Sometimes when I google for installation instructions (for a gem, plugin whatever) I come across with blog posts containing old and out of dated installation instructions (old versions for example).
Why not create a pastie like engine for people to create and search for the most updated instructions? This engine should be smart enough to get a URL/svn/git/FTP address and fetch the latest file for each entry.
Of douse, a widget for bloggers should be mandatory.
I don't have the time to do it alone, if anyone feels like picking up this glove with me, just tell me.
Ruby On Rails and a Conning Israeli entrepreneur
Free idea: pastie like installation instructions
Periodical AJAX requests and Session Expiry
My current applicaiton requires a session expiry feature, so fat, pretty ordinary, i decided to use the limited sessions plugin, as i always do.
The problem came up when i found out, that sessions aren't being released after the desired time had passed.
What was the problem?
first we will need to understand how rails uses sessions, basically it's something like that
- session is retrieved from whereever it is. (ActiveRecordStore,CookieStore...)
- Controller#Action is being preformed
- Session is saved
Solution
In my application.rb, i have a before_filter which updated the session with user_id in order to relate the session to the user, i migrated another field into the session table so i can keep my own track over changes, named it 'last_updated' like that
class AddCustomUpdateDateToSessions < ActiveRecord::Migration def self.up add_column :sessions, :last_updated, :datetime end def self.down remove_column :sessions, :last_updated end endand changed the application.rb method to update the new field, instead of the 'updated_at' field, and prevented my AJAX requests to update to the session, no need.
before_filter :update_session def update_session unless request.xhr? if logged_in? if session.model.user_id.nil? session.model.update_attribute(:user_id, current_user.id) end session.model.update_attribute(:last_updated, DateTime.now) end end end(yeah, i know it can be done better.) i also needed to change all the 'updated_at' references to 'last_updated' field inside the plugin's limited_sessions.rb, in order to completely take control over the session update flag. now it's working and my ajax calls do not cause the expiry interval to expire.
attachment_fu and minimagick resize bug
In one of my latest projects i was using the attachement_fu plugin to handle image uploads.
The attachement_fu plugin enables the option to select an image processor engine between ImageScience, Rmagick add MiniMagick:
- Rmagick
Kinda bulky, leaking but fully functional - MiniMagick
Simple version of Rmagick, simple and small. - ImageSceince
Small, nice, and quick.
sudo gem install mini_magick
and added a specific :processor option to my Logo model.
has_attachment :content_type => :image,
:storage => :file_system,
:max_size => 2.megabytes,
:resize_to => 'x100',
:processor => :mini_magick
but when i was uploading an image, i was was expecting it to be resized based on the options i specified in the model. imagine how surprised i was when i found out that the image is not being resized.
checking the log found the following error:
Exception working with image: ImageMagick command (identify "/var/folders/Bv/Bvbna8-OH548ZWju8naF4U+++TI/-Tmp-/minimagick11451-0") failed: Error Given 32512
Googling it didn't help me much (except from finding other people with the same problem), so i decided to switch to ImageScience instead (RMagick is out of the question of course).
sudo gem install image_science
this will include all most all dependencies, the freeImage dependency is also needed.
us mac/darwin users can simply use port:
sudo port selfupdate
sudo port install freeimage
now change the processor in the model
has_attachment :content_type => :image,
:storage => :file_system,
:max_size => 2.megabytes,
:resize_to => 'x100',
:processor => :image_science
and now it's working. yeah!.
Skype Weblinks - Call a skype user or send an IM message in a html page
When the user has installed Skype, one can open Skype directly from a webpage. The protocol is skype:skypename - and some other optional flags.
The example will use my skype username, but i am sure you will be able to get the hang of it.
Call a skype user
The optional flag is call - to force Skype to call somebody. It can also be left out - but if the user changed the default behavior to e.g. chat - Skype will open an IM session instead. So - go the save way and use the call - flag.
Example: call a Skype user
<a href="skype:eladmeidar?call">Call</a>
Send an Skype IM message to a Skype user
The optional flag is chat. See the example below to see the usage in a html page.
Example: send a chat message to a Skype user
<a href="skype:eladmeidar?chat">Chat</a>
Send a voicemail to a Skype user
The optional flag is voicemail - as you might have guessed.
Example: send a voicemail to Skype user
<a href="skype:eladmeidar?voicemail">Voice mail</a>
Create a Skype conference call with multiple users
This is as easy as the examples above - just more usernames before the "?" and call as flag.
Example: create a Skype conference call
<a href="skype:eladmeidar;otherfriend123?call">call 2 friends</a>
Create a conference chat with multiple users
Same as conference call - with chat as flag.
Example: open a conference chat with multiple users - in html
<a href="skype:eladmeidar;otherfriend123?chat">Chat with 2 friends </a>
i am now working on a rails plugin to implement this automated use for Rails applications
Rails 2.2: Getting Started link list
General Documentation and Guides
From railsinside.com
Rails 2.2 Release Notes - A very solid set of release notes for 2.2 with basic coverage of the new features (with short code examples and links) as well as a list of deprecated features. They were compiled by Ruby / Rails Inside's very own Mike Gunderloy!
Upgrading RubyGems to 1.3.x - Depending on your setup, Rails 2.2 may demand that you upgrade to RubyGems 1.3.x. This is not as easy as it might usually be, however. Mike Gunderloy gives some tips in case you get stuck.
Rails Security Guide - Steer clear of security issues in your Rails 2.2 applications by reading the Ruby on Rails Security Guide. Who said Rails has poor documentation? This is incredible!
Rails 2.2 Screencast - Gregg Pollack and Jason Seifer of Rails Envy put together a very solid Rails 2.2 screencast. It costs $9, but it covers a lot of ground over 44 minutes - learn about etags, connection pooling, new enumerable methods, new test helpers, and more.
Rails 2.2 - What's New - In association with EnvyCasts, Carlos Brando and Carl Youngblood present Rails 2.2 - What's New, a 118 page PDF covering all of the changes and additions to Rails 2.2. It's available in a package deal with the screencast (above) too.
InfoQ's Glance - InfoQ's Mirko Stocker takes a quick glance at some of Rails 2.2's new features.
New Features
Thread Safety - Rails 2.2 is now "thread safe." In October, Pratik Naik wrote a summary of why this is a big deal as well as some gotchas (basically, don't use class variables, use mutexes, etc.) Charles Nutter has also written What Thread-safe Rails Means which answers several pertinent questions.
Internationalization - The Rails Internationalization effort has its own homepage at http://rails-i18n.org/ which features lots of links to how-tos, tips, documentation, and demos. They also have a Google group / mailing list where you can get help, make suggestions, etc.
Basic Language Internationalization - It's a little old, but Simple Localization in Rails 2.2 gives a very quick, code-driven example of how basic internationalization works in Rails 2.2 (some of the set up is easier now, but it mostly applies).
Localization / Internationalization Demo App - Clemens Kofler has put together a demo app that shows off some of Rails 2.2's internationalization and localization features. If being knee deep in code is the best way for you to learn, jump in!
Layouts for ActionMailer - As of Rails 2.2, you can now use layouts in your ActionMailer views.
Connection Pooling - The connection pooling in Rails 2.2 allows Rails to distribute database requests across a pool of database connections. This can cause less lockups. In collaboration with a non-blocking MySQL driver, serious performance increases could result in certain situations.
Specify Join Table Conditions with Hashes - Do you need to run a find (or similar) query across a join? Now you can just specify the conditions for the joined tables in a hash, much like local tables conditions!
Limited Resource Routes - You can now limit map.resources to creating certain methods. For example, you might not want destroy or index methods - you can now specify these with :only and :except.
Memoization - Stop rolling your own memoization in Rails apps. Clemens Kofler demonstrates Rails 2.2's newly rolled-in memoization features. It's just a single method! If you have a view that calls on a calculated attribute often, this will give you some serious performance gains.
Custom Length Tokenizer for Validations - You can now specify a tokenizer of your own construction for validates_length_of validations.
Array#second through Array#tenth - If you're a bad programmer, you can now demonstrate it to the world by using the new Array#second, Array#third, Array#fourth, and so forth, methods. I've put it in my calendar to look for open source Rails apps using Array#seventh in six months time and to call them out on Rails Inside ;-)
Note: This list only takes into account some of the new features in Rails 2.2. There are a lot more! Read the release notes and the Rails 2.2 - What's New PDF to get the full picture.
Miscellaneous
restful-authentication-i18n - Want an authentication plugin for Rails 2.2 that supports internationalization? Take a look at result-authentication-i18n!
Barebones Apps - Check out Rails Inside's 7 Barebones Rails Apps to Kick Start Your Development Process.
Deploying on JBoss - You can now easily deploy a Rails app to a JBoss server. With Rails 2.2's significantly improved JRuby support, this makes rolling out Rails apps in the enterprise a breeze!
Installing Rails on Ubuntu Hardy Heron - Simon St Laurent has put together two Rails useful installation videos. One for servers, and one for the desktop.
REST for Rails 2 - Are you still in Rails 1.x land or not using REST at all? Would you like to? Geoffrey Grosenbach has put together a screencast showing you how it should be done.
A Better Rails Logo - The Rails Logo (as used at the head of this post) was created by Kevin Milden and is distrubuted under the BY-ND Creative Commons Licence. i personally like the older one.
Rails 2.2: Quick Feature list
Rails 2.2 was just released, and ryan strieks again with a great post.
let’s take a peek at some of the major new features (as determined by yours truly – feel free to pipe up with features that I’ve missed).
Rails 2.2.2 requires rubygems v 1.3.1, so before you upgrade make sure to do a sudo gem update --system
.
Rails 2.2 Features
- :except and :only Routing Options
- Better Conditional GET Support
- Connection Pools (Rails 2.2 is thread-safe!)
- Mailer Layouts
- Shallow Routes
- Simpler Conditional Get Support, And Even Better Conditional GET Support
- Standard Internationalization Framework
- Get Your Metaclass
- Easy Memoization
- Easy Join Table Conditions
- Custom Length Validation Tokenizer
- Collection Partial Variable Naming
i am personnalt still on 2.1, and i don't think i will upgrade at this moment, but some features look very-needed in fact.
Printer Friendly Pages
CSS is a great new tool for web designers. Now it is more widely supported, and browser-makers are sticking to the
W3C standards, we can begin to explore the possibilities it has offered us since the 90s but that we could not make
use of.
CSS allows you to separate your design from your markup, making maintenance infinitely easier, and your site more
likely to work in different devices, both of which are definite pluses. It also, however, allows you to tell a page to
display a different way depending upon what device is being used to render it.
This is achieved using the "media" attribute of the "<link>" or "<style>" tags, like so:
There are several media types available to you - all, aural, braille, handheld, print, projection, screen, tty, tv - and a
list of available media types with more detail is available from W3C (where you can find out more about media
groups, as well).
With such a range of options, you can, if you like, create one HTML document that will display exactly as you like
depending entirely upon which device is being used to view it. You can hide large images from PDAs, you can specify
seperate font styling for overhead projectors - you can write your site so that it works well with the technology at the <link media="screen" href="style.css" type="text/css">
<style media="screen" type="text/css">
user's disposal. And one very useful side effect of this is that you can specify an entire print style without the need
for copies of every page of your content, like the following, that will use the "normal.css" style sheet for screens, and
the "printer.css" style sheet when printing.
<link media="screen" href="normal.css" type="text/css">
<link media="print" href="printer.css" type="text/css">
For print styling, there are a lot of factors to consider. Are many users going to want to print out your header? No.
But do you want your logo on the page? Yes. Is the navigation worth printing? No. Each item on a web page serves a
purpose, and on paper, that may not be worth much.
It is best, then, to start at the beginning. With an unstyled HTML document. Pick a page to start working on, and
remove all styles from it. With an unformatted page in front of you, it should be pretty easy to see what needs styling
and how, but don't rush in. There's a lot to think about.
FONTS
Fonts are very different on the web to print. Sans-serif fonts are, allegedly, the best to use on the web. Serif fonts
are, apparently, better on paper. So first things first, set your font to a serif font you like (personally, I like Georgia
for Windows). As with picking all fonts, make sure you provide alternatives for those without the first-choice font.
Font sizing is a bit different on paper too. It's usually best just to leave this to the default, but if you want to specify a
font, make sure you run plenty of tests to make sure it's easily legible.
* {
font-family: Georgia, "Times New Roman", Times, serif;
font-size: 12pt;
}
LINKS
Links are not going to be a lot of use on paper, by their very nature. But that does not make them useless.
a {
color:#000000;
text-decoration: underline;
}
a:after {
content: " ( "attr(href)" )";
}
The above, if added to a printer style sheet, will make all links black and underlined, and add the address of the link
in brackets afterwards, meaning that if a user did want to visit any site linked to from that page at a later date, they
can.
SPACING
In print, margins and spacings are as important as with the web. There is no harm in adding in a little space
between words and lines, and a decent gap around the edges, if it will make a print-version easier to read.
HIDE USELESS STUFF
You can hide elements in this style sheet easily enough, using display none. This will save some space on the paper,
and reduce useless junk on the page, something the user will be very grateful for. Elements worth hiding are usually
form elements, some images, marquees, and flash content. If you are unsure, print the page and then work through
that page deciding what is useful and what is not.
body {
padding: 10%;
line-height: 1.2;
word-spacing: 1px;
}
COLORS
Print colors will show up very differently to screen colors, especially as a high proportion still have black and
white printers. Even those who don't will be grateful to you if you keep the proportion of color to a minimum,
simply because of the expense.
Highlight and emphasize things using italics, underlining, boldness and size in your print style sheet. Remove all references to colors where you can, save to set colors to black.
PAGE ADDRESS
You may want to add the address of the page itself to the page, and hide it, except when the page is printed, so that
the user can return to your page later if they wish.
TELL THE USER
A user may see a page without a "printer friendly" link and print anyway, but they may be put off. Try and add a note
to your page explaining that the page they are now viewing is printer friendly, and encouraging them to print it.
Rails date Range
Holy Shmoly! Sometimes Rails and ruby just blows me away,
Let’s assume you are trying to find a bunch of records between a time frame.
class Call < ActiveRecord::Base
named_scope :by_month, lambda { |d| { :conditions => { :date => d.beginning_of_month..d.end_of_month } } }
end
simply specify a range
and running (the named_scope )
this_months_calls = Call.by_month Date.today
And Rails will return all the calls that were recorded during the current month. Love it.
Git vs. Mercurial
Personally I'm more a fan of Git with the main reason being: Branching.
As far as I know Git still has problems handling Windows which is something Mercurial has never had to worry about. But since i am not a windows user (lover, or any other positive opinion holder), i don't really care.
Git
Pros
Lots of features
More hosting options.
Faster - slightly.
GitHub.
Solid local branch support.
Cons
Poor Windows support
Rubbish logo
Silly, long SHA1 hash of revisions.
You need to keep 'packing' the repo
History fiddling.
Mercurial
Pros
Portable
Its built with Python
Easily extensible using Python
Cool logo
Better documentation.
Local integer revision numbers on top of the SHA1
No need to keep packing/optimising.
Smaller repo footprint.
Better patch support (bundles and such)
Easy to learn.
Cons
Smaller feature set.
Few 3rd party hosting options.
Fewer 3rd party apps and plugins.
Smaller community.
Slower development.
Who Uses What
Mercurial
Mozilla
OpenSolaris
Aptitude
Netbeans
Dovecot
Git
Ruby on Rails Core
Linux Kernel
Google Android
Beryl
Fedora
Who Needs What
Mercurial is great for people who want to dive into distributed version control and get busy. Its clean, fast and easy to learn.
Git is for those who wish to fully exercise the benefits of source control and avoid the down's often happen when collaborating code.
goosh.org: a Google Command Line!
http://goosh.org/ is a command line interface for Google and some other related services such as wikipedia and YouTube.
Goosh (Google Shell) is a google-interface that behaves similar to a unix-shell.
You type commands and the results are shown on this page.
goosh was written by Stefan Grothkopp <grothkopp@gmail.com>
it is NOT an official google product!
Google Chat Video
Google is rolling out video and voice capabilities for the chat function that is embedded in the Gmail interface. It's a bare-bones voice and video-conferencing service, but it's simple to install and use and is a very good addition to Gmail.
It's no Skype, though. Gmail Video and Voice, as it's called, can't connect to the plain phone network, as Skype's paid service can. And there are plenty of other optional features missing, like a voice call recorder.
MochaUI: Javascript User interface library
MochaUI is a web applications user interface library built on the Mootools JavaScript framework.
Uses:
Web Applications
Web Desktops
Web Sites
Widgets
Standalone Windows and Modal Dialogs
Get it and watch demos here
CSS Graphs Helper for Ruby on Rails
source: http://nubyonrails.com/pages/css_graphs
A Ruby on Rails helper for making simple graphs. The graphs use only CSS/HTML (and an optional gradient image).
Vertical bar graphs, horizontal bar graphs, and complex bar graphs with an image marker.
Install:
./script/plugin install http://topfunky.net/svn/plugins/css_graphs
Generate:
# No arguments are needed ./script/generate css_graphs
Examples:

Find out more on the source @ Nuby on Rails
Common RJS mistake/error on Ruby on Rails
When you use some of your ajax helpers, such as link_to_remote, with the :update parameter in order to update a specific DOM element AND you specify page.replace_html or any other RJS syntax that handles other DOM ID, it will return the result expected, nothing will be updated on the :update pointer DOM ID.
Simply choose one, if you use :update, return an html or render a partial in your action instead of rendering RJS.
If you require multiple changes, use RJS and no :update parameter.
bye bye pdfetch.com
I decided to shutdown pdfetch.com, although it was a nice idea, i don't really have the time to take care of it.
thank you all the 300,000 users who downloaded a pdf from my site, if anyone wants the code, just as for it in the comments.
A little bit abot Git
Distributed Nature
Git was designed from the ground up as a distributed version control system.
In a distributed version control software like Git every user has a complete copy of the repository data stored locally (a.k.a a local working copy), thereby making access to file history extremely fast, as well as allowing full functionality and access when disconnected from the network. It also means every user has a complete backup of the repository. If any repository is lost due to system failure only the changes which were unique to that repository are lost. If users frequently push and fetch changes with each other this tends to be an incredibly small amount of loss, if any at all.
In a centralized VCS like Subversion only the central repository has the complete history. This means that users must communicate over the network with the central repository to obtain older versions for a file, Backups must be maintained independently and if the central repository is lost due to system failure it must be restored from backup and all changes since that last backup are likely to be lost. (Depending on the backup policies).
Access Control
Due to being distributed, you inherently do not have to give commit access to other people. Instead, you decide when to merge what from whom.
Branch Handling
Branches in Git are a core concept used everyday by every user. In Subversion they are almost an afterthought and tend to be avoided unless absolutely necessary.
The reason branches are so core in Git is every developer's working directory is itself a branch. Even if two developers are modifying two different unrelated files at the same time it's easy to view these two different working directories as different branches stemming from the same common base revision of the project.
So than Git:
- Automatically tracks the project revision the branch started from.
- Knowing the starting point of a branch is necessary in order to successfully merge the branch back to the main trunk that it came from.
- Automatically records branch merge events.
- Merge records always include the following details:
- Who performed the merge.
- What branch(es) and revision(s) were merged.
- All changes made on the branch(es) remain attributed to the original authors and the original timestamps of those changes.
- What additional changes were made to complete the merge successfully.
- Any changes made during the merge that is beyond those made on the branch(es) being merged is attributed to the user performing the merge.
- When the merge was done
Why the merge was done (optional; can be supplied by the user).
- Automatically starts the next merge at the last merge.
- Knowing what revision was last merged is necessary in order to successfully merge the same branches together again in the future.
Performance
Git is extremely fast. Since all operations (except for push and fetch) are local there is no network latency involved to:
- Perform a diff.
- View file history.
- Commit changes.
- Merge branches.
- Obtain any other revision of a file (not just the prior committed revision).
- Switch branches.
Space Requirements
Git's repository and working directory sizes are extremely small when compared to SVN.
Google Gmail search tokens
The amazingly simple search within Gmail (Google Apps) enables the option to specify a token in order to make your life a little easier.
Operator | Definition | Example(s) |
---|---|---|
from: | Used to specify the sender | Example - from:elad Meaning - Messages from Amy |
to: | Used to specify a recipient | Example - to:elad Meaning - All messages that were sent to David (by you or someone else) |
subject: | Search for words in the subject line | Example - subject:dinner Meaning - Messages that have the word "dinner" in the subject |
OR | Search for messages matching term A or term B* | Example - from:amy OR from:david Meaning - Messages from Amy or from David |
- (hyphen) | Used to exclude messages from your search | Example - dinner -movie Meaning - Messages that contain the word "dinner" but do not contain the word "movie" |
label: | Search for messages by label* *There isn't a search operator for unlabeled messages | Example - from:amy label:friends Meaning - Messages from Amy that have the label "friends" Example - from:david label:my-family |
has:attachment | Search for messages with an attachment | Example - from:david has:attachment Meaning - Messages from David that have an attachment |
list: | Search for messages on mailing lists | Example - list:info@example.com |
filename: | Search for an attachment by name or type | Example - filename:physicshomework.txt Example - label:work filename:pdf |
" " | Used to search for an exact phrase* *Capitalization isn't taken into consideration | Example - "i'm feeling lucky" Example - subject:"dinner and a movie" |
( ) | Used to group words Used to specify terms that shouldn't be excluded | Example - from:amy(dinner OR movie) Example - subject:(dinner movie) |
in:anywhere | Search for messages anywhere in Gmail* *Messages in Spam and Trash are excluded from searches by default | Example - in:anywhere movie Meaning - Messages in All Mail, Spam, and Trash that contain the word "movie" |
in:inbox in:trash in:spam | Search for messages in Inbox, Trash, or Spam | Example - in:trash from:amy Meaning - Messages from Amy that are in Trash |
is:starred is:unread is:read | Search for messages that are starred, unread or read | Example - is:read is:starred from:David Meaning - Messages from David that have been read and are marked with a star |
cc: bcc: | Used to specify recipients in the cc: or bcc: fields* *Search on bcc: cannot retrieve messages on which you were blind carbon copied | Example - cc:david Meaning - Messages that were cc-ed to David |
after: before: | Search for messages sent during a certain period of time* *Dates must be in yyyy/mm/dd format. | Example - after:2004/04/16 before:2004/04/18 Meaning - Messages sent between April 16, 2004 and April 18, 2004.* *More precisely: Messages sent after 12:00 AM (or 00:00) April 16, 2004 and before April 18, 2004. |
is:chat | Search for chat messages | Example - is:chat monkey Meaning - Any chat message including the word "monkey". |
Ruby on Rails and Oracle on Mac Os Leopard
Overview
The nightmare is over.
Just until the latest Oracle libraries update (finally released a X86 library pack for mac) it was nesecerry to use 2 versions of ruby, a universal and a ppc version. Sadly, when running PPC, the benchmarking were terrible and it had some very annoying freezes and other stuff that would simple make you want to jump off the roof.
BUT! (:) ) times had changed, Oracle (as mentioned) released an X86 Intel compatible library pack for MacOs users and therefore ended my misery,
Woohoo! That makes the entire process of connection Ruby on Rails and oracle on Leopard about as 100 times less complicated than before, so I’ve posted it here to let everyone enjoy.
I assumes that you’re using Rails 2.0 or greater (Why not really?).
IMPORTART!!!
If you already connected Oracle and Ruby on Rails using the old way, please preform the "Cleanup" step first.
Oracle Libraries
The new Intel Mac versions are available from the Oracle downloads site. Install them in /Library/Oracle/.
You can do side-by-side installations in folders with whatever names you want, since apps find them by using the $ORACLE_HOME environment variable (and it’s friends). I’ve got mine in /Library/Oracle/instantclient_10_2.
Also make sure that you’ve got the files required to run sqlplus and the sdk along with the basic. You can drop those in the same directory.
Symlink the libraries
In the directory where you’ve installed the instant client, run this:
ln -s libclntsh.dylib.10.1 libclntsh.dylib
Set the environment variables correctly
You’ll probably want to put these lines in your /etc/profile , but they also must be run from the command line to take effect (you can also "source /etc/profile"):
export ORACLE_HOME=/Library/Oracle/instantclient_10_2 <= Change to your library!
export TNS_ADMIN=$ORACLE_HOME
export LD_LIBRARY_PATH=$ORACLE_HOME
export DYLD_LIBRARY_PATH=$ORACLE_HOME
export PATH=$PATH:$ORACLE_HOME
Oracle! giddie up!
First you'll need to install the Active Record Oracle adapter,
sudo gem install activerecord-oracle-adapter --source http://gems.rubyonrails.org
which is how ActiveRecord deals with Oracle.
It doesn’t, however, install the Ruby oci8 driver, which is how Ruby talks to Oracle (yeah, annoying).
Important!!!
Have you installed the Oracle Instant Client SDK ?
good.
Get the lastest the oci8 library. Download it and unpack the file in the finder: it should unzip into ~/Downloads/ruby-oci8-x.x.x.
Now we can finish configuring the environment before we compile the library.
cd ~/Downloads/ruby-oci8-x.x.x
export SQLPATH=$ORACLE_HOME
export RC_ARCHS=i386
ruby setup.rb config
make
sudo make install
oh joy, scrolling lines of doom will pass in front of you and hopefully you'll see no errors and burst into tears.
Show me!
At this point, we’re almost done. Let's see it working.
irb
require 'oci8'
==> true
or
irb
require 'rubygems'
==> []
require 'oci8'
==> true
Configure your database.yml
In your database.yml, use the following to make it work:
development:
adapter: oracle
database: your_instance_name
username: your_user_name
password: your_password
The database name = > comes straight out of your tnsnames.ora file. You don’t need to specify any other connection information in database.yml, since the tnsnames.ora file has everything you need.
if you are using the oracle Express edition, it should look something like that.
development:
adapter: oci
host: //db_hostname:db_port/xe <== Oracle port is usually 1521
username: username
password: password
cursor_sharing: similar
prefetch_rows: 100
note: last 2 lines are some tweaking for a better Oracle performance.
Cleanup: Fix the ruby_fat and ruby_ppc setup
if you have installed Oracle libraries using the old way, you'll be happy to remove the mess it made out of your ruby installation and happily, it’s quite simple.
Just remove the ruby_ppc files, and the symlinks to them (called ruby, and rename ruby_fat as ruby:
cd /usr/bin <== or wherever you put them.
sudo rm ruby
sudo rm ruby_ppc
sudo mv ruby_fat ruby
and
cd /System/Library/Frameworks/Ruby.framework/Versions/1.8/usr/bin
sudo rm ruby
sudo rm ruby_ppc
sudo mv ruby_fat ruby
Then, you should also remove the 2 management scripts:
sudo rm /usr/bin/ppc_ruby.sh
sudo rm /usr/bin/fat_ruby.sh
And that’s enough for the cleanup.
Rails Validations - Overview
i keep forgetting that, so i post it here for me and everyone else.
Here is the list of validations which you can perform on user input:
validates_presence_of:
Following check that last_name and first_name should be filled and should not be NOLL.
validates_presence_of :firstname, :lastname |
validates_length_of:
Following example shows various validations on a single filed. These validations can be performed separately.
validates_length_of :password, |
validates_acceptance_of:
Following will accept only 'Y' value for option field.
validates_acceptance_of :option |
validates_confirmation_of:
The fields password and password_confirmation must match and will be used as follows:
validates_confirmation_of :password |
validates_uniqueness_of:
Following puts a condition for user_name to be unique.
validates_uniqueness_of :user_name |
validates_format_of:
Following validates a that given email ID is in a valid format. This shows how you can use regualr expression to validatea filed.
validates_format_of :email |
validates_numericality_of:
This validates that given field is numeric.
validates_numericality_of :value |
validates_inclusion_of:
Following checks that passed value is an enumeration and falls in the given range.
validates_inclusion_of :gender, |
validates_exclusion_of:
Following checks that given values does not fall in the given range.
validates_exclusion_of :age |
validates_inclusion_of:
Following checks that given values should fall in the given range. This is opposite to validates_exclusion_of.
validates_inclusion_of :age |
validates_associated:
This validates that the associated object is valid.
Options for all Validations:
You can use following options alongwith all the validations.
:message => 'my own errormessage' Use this to print a custom error message in case of validation fails.
:on => :create or :update This will be used in such cases where you want to perform validation only when record is being created or updated. So if you use :create then this validation work only when there is a create opration on database.
Check the following link for more detail on Validations.
TextMate Bundle of Bundles: getBundle
From Macroomates
There are 124 bundles for TextMate where only 36 are included by default. Until recently, the way to get more bundles has been to install subversion and then do a checkout of the bundles needed (from the shell.) or download a .tmbundle file.
Now there is a much easier way: the GetBundle bundle by Sebastian Gräßl. All you need to do is download and double click it to get an “Install Bundle” command which you can invoke from inside TextMate (hint: use ⌃⌘T and enter “install”.)
There is also an “Update all Bundles” command which you can use to update your custom installed bundles to the latest version. A future version of the bundle is likely to offer a launchd Daemon that you can install to have updating taken care of automatically.
All bundles installed via the GetBundle end up in ~/Library/Application Support/TextMate/Pristine Copy/Bundles.
Get it here, since the original repo isn't working.
Twiters Around
About Me
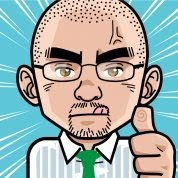
- Elad Meidar
- I am a web developer for more than 9 years, managed, cried, coded, designed and made money in this industry. now trying to do it again.
Blog Archive
-
▼
2008 (91)
-
▼
November (21)
- Free idea: pastie like installation instructions
- Periodical AJAX requests and Session Expiry
- attachment_fu and minimagick resize bug
- Skype Weblinks - Call a skype user or send an IM m...
- Rails 2.2: Getting Started link list
- Rails 2.2: Quick Feature list
- Printer Friendly Pages
- PING.fm
- Rails date Range
- Git vs. Mercurial
- goosh.org: a Google Command Line!
- Google Chat Video
- MochaUI: Javascript User interface library
- CSS Graphs Helper for Ruby on Rails
- Common RJS mistake/error on Ruby on Rails
- bye bye pdfetch.com
- A little bit abot Git
- Google Gmail search tokens
- Ruby on Rails and Oracle on Mac Os Leopard
- Rails Validations - Overview
- TextMate Bundle of Bundles: getBundle
-
▼
November (21)
Labels
- 1.9 (1)
- 2.0 (1)
- 2.2 (1)
- 2.3 (1)
- 2.x (1)
- accessibility (2)
- account (1)
- actionmailer (1)
- activerecord (3)
- adsense (1)
- affiliate (1)
- ajax (3)
- amazon (4)
- analytics (1)
- api (3)
- application (1)
- array (1)
- associations (1)
- attachment_fu (1)
- autocomplete (1)
- aws (2)
- blog (4)
- books (4)
- boolean (1)
- browser (8)
- browsers (3)
- bugs (4)
- buzz (1)
- callback (1)
- callbacks (1)
- caller (1)
- capistrano (1)
- chronic (1)
- class (2)
- classes (1)
- classifieds (1)
- client (1)
- coding (2)
- collboration (1)
- console (2)
- convert (1)
- core (2)
- core. object (1)
- cost (1)
- css (7)
- database (10)
- date (3)
- dating (1)
- db (2)
- debug (2)
- deploy (5)
- deployment (3)
- design (11)
- development (5)
- dojo (1)
- eager (1)
- ec2 (2)
- effects (1)
- elad (2)
- email (3)
- engine (1)
- engineyard (1)
- english (1)
- entrepreneur (1)
- environment (2)
- erd (1)
- error (1)
- example (1)
- expire (1)
- explorer (1)
- extention (1)
- extra (2)
- facebook (2)
- fast (1)
- file (1)
- files (1)
- firefox (4)
- fixtures (1)
- font (1)
- form (1)
- framework (16)
- free (6)
- funny (2)
- gem (7)
- gems (2)
- git (5)
- globalize (2)
- gmail (3)
- google (8)
- graphes (2)
- graphics (1)
- guides (4)
- hacks (1)
- hosting (1)
- html (1)
- http (1)
- ie (5)
- ie8 (1)
- image (2)
- indomite (1)
- install (1)
- iphone (1)
- israel (2)
- javascript (19)
- JQuery (10)
- js (3)
- legacy (1)
- leopard (1)
- lib (1)
- library (3)
- links (2)
- list (1)
- loading (1)
- log (1)
- logger (2)
- logging (1)
- love (1)
- mac (4)
- mail (2)
- manuals (1)
- mapping (1)
- mass (1)
- mephisto (3)
- mercurial (1)
- meta (1)
- metaprogramming (3)
- microsoft (1)
- migrations (2)
- minimagick (1)
- mistake (1)
- mistakes (1)
- model (1)
- money (4)
- mootools (4)
- move (1)
- movies (2)
- music (1)
- mvc (2)
- mysql (2)
- native (1)
- nested (1)
- network (1)
- new (1)
- newsletter (1)
- num_to_english (1)
- numbers (2)
- object (1)
- observer (1)
- ohad (1)
- open (1)
- operators (1)
- optimization (4)
- oracle (4)
- overload (1)
- parse (1)
- pdf (4)
- php (3)
- plugin (11)
- plugins (7)
- programming (4)
- programs (1)
- projects (5)
- prototype (1)
- queries (1)
- rails (50)
- rails 2.0 (10)
- rails 2.1 (6)
- rake (2)
- rants (1)
- record (1)
- reflections (1)
- release (3)
- rendering (1)
- reset (1)
- resize (1)
- rest (1)
- rjs (1)
- ror (1)
- routes (3)
- routes.rb (2)
- ruby (49)
- ruby on bells (2)
- ruby on rails (28)
- ruby-debug (1)
- s3 (2)
- salary (1)
- scaling (2)
- schema (2)
- screencasts (1)
- script (4)
- scripts (1)
- search engine optimization (1)
- security (3)
- seeking alpha (1)
- select (1)
- sendmail (1)
- seo (2)
- sequencer (1)
- sessions (1)
- share (2)
- shell (1)
- site (3)
- site map (1)
- sites (1)
- skype (1)
- social (2)
- source (1)
- source control (4)
- sprites (1)
- sql (1)
- stand alone (1)
- standards (1)
- startup (6)
- storage (2)
- subversion (4)
- svn (1)
- team (1)
- technology (1)
- testing (1)
- textmate (1)
- thread (1)
- time (1)
- tips (45)
- tools (5)
- tracking (1)
- tricks (36)
- tutorials (5)
- ui (4)
- uml (1)
- update (1)
- upgrading (2)
- url (1)
- usability (2)
- user interface (7)
- validations (1)
- vector (1)
- vote (1)
- web (9)
- web services (2)
- web2.0 (4)
- webistrano (1)
- wire (1)
- wwr (1)
- xhtml (1)
- yahoo (1)
- yui (1)
- רובי (8)
- רובי און ריילס (7)