Yaiii!
finally.
If you are here it means that you have an old link, or got redirected by a old RSS entry, please refer to my new blog "Emphasized insanity" and update your RSS subscription.
Ruby On Rails and a Conning Israeli entrepreneur
Moved to Mephisto
Rails Nested Resources Tutorial
Preface
The best way to get started with Rails 2.x nested routing and routing at all, is to read the official Rails Routing guide at the Rails Guides website.Rails Nested Routes/Resources
The rails nested routes/resources were indroduced at Rails 1.2 as part of the RESTful approach that was adopted by the Rails core members.Nested resources allowed urls like:
/tasks/?project_id=1
To have a bit more sexy/RESTful look:
/projects/1/tasks
Let’s use this example to make things a little more clear, we will use 2 models, Project and Task
# /app/models/project.rb
class Project < ActiveRecord::Base
has_many :tasks
end
# /app/models/task.rb
class Task < ActiveRecord::Base
belongs_to :project
end
Setting up routes.rb
Creating a Nested Resource
:has_many keyword
The easiest way to create a nested route, is to use the :has_many keyword like that:# /config/routes.rb
map.resources :projects, :has_many => :tasks
# and the correspondent task resource
map.resources :tasks
Adding the second routes, that defines a RESTful route to :tasks, depends if you would like to allow an access to the Task resource, without the project context, this is not a must.
Block
You can also specify the sub-resources in a blockmap.resources :projects do |project|
project.resources :tasks
end
Singular Resources
Just the same:map.resources :projects do |project|
user.resource :design_document
end
Routes Helpers
Run:$ rake routes
to see what kind of routes do you have in your application, you can pipe UNIX’s grep command (”| grep xxx”) to filter the results:
$ rake routes | grep project
A basic map.resources :projects will produce:
events GET /projects {:controller=>"projects", :action=>"index"}
formatted_projects GET /projects.:format {:controller=>"projects", :action=>"index"}
POST /projects {:controller=>"projects", :action=>"create"}
POST /projects.:format {:controller=>"projects", :action=>"create"}
new_project GET /projects/new {:controller=>"projects", :action=>"new"}
formatted_new_project GET /projects/new.:format {:controller=>"projects", :action=>"new"}
but a Nested route, like we defined before will produce:
project_tasks GET /projects/:project_id/tasks
new_project_task GET /projects/:project_id/tasks/new
edit_project_task GET /projects/:project_id/tasks/:id/edit
project_task GET /projects/:project_id/tasks/:id
very nice.
Singular Nested Route Helpers
(from the example above)
/projects - list all projects
/projects/1 - show a single project
/projects/1/design_document - a project’s design document
Using the routing helpers
Since we now have another resource in context when we want o use the new helpers, we need to include that resource instance as a paramter:
new_project_task(@project)
# or when both resources are required
edit_project_task(@project, @task)
Forms
I'll assume you use form_for in your forms, it will make the usage of nested resources a lot easier than to work with plain HTML or form_tag.
The regular form we know of form_for, receives one instance as the form object:
<% form_for(@project) do |f| %>
...
<% end %>
But with nested resources, we'll pimp it up a little bit:
<% form_for([ @project, @task ]) do |f| %>
...
<% end %>
Note the instances array, that specifies the objects we need in our form when we deal with nested resources
Conclusion and some Gotchas.
Using nested resources and routes is the right thing, URLs are clear, and code is readable. but:
# You should not implement nested resources of more than 2 levels.
# Setting up pagination support (?page=3) kind of breaks the RESTful approach.
# The railscast about Nested Resources.
# Using RESTful ajax calls, a great lib by dfr|work (#rubyonrails).
Raphael: Amazing vector graphics Javascript Libraty
Raphaël is a small JavaScript library that should simplify your work with vector graphics on the web. If you want to create your own specific chart or image crop and rotate widget, for example, you can achieve it simply and easily with this library.
Raphael Supports Firefox 3.0+, Safari 3.0+, Opera 9.5+ and Internet Explorer 6.0+ which is pretty awesome and allows you to do almost any graphic task you have in mind, just check the demos.
Raphael weighs about 110kb uncompressed, which is kind of an issue i think.
Get it here at the Raphael Homepage
Storing Files in Ruby on Rails
I finished my post before reading theirs and didn't add things Koz wrote and i didn't, so don't blame me in stealing or something. :)
I Know storing files in a database is usually a bad idea, but i still thought about the face that i was yet to see a propar guidance on how to handle files (using the correct practices of course).
Plugins
Attachement_fu - The older brother, pretty nice, better than the late file_column.
PaperClip - An "updated" attachement_fu, some people say it's better than attachement_fu because it is actually working. There is a lot more work being done on this plugin now a days, so it's better to relay on it rather than on attachment_fu.
Storing your files.
Local File System
This option can only work out for you, if you are running your app on a single server, simply set one of the plugins to store the uploaded files whereever you want, and that's it.
You can also setup a network folder if you'd like and imitate a local folder across a number of servers, but i's the same.
What you do need to be careful from, is a situation where you are saving too many files into a single folder, which will cause mainly for a slow file lookup.
Try to refine you directory structures for your upload root in order to avoid such a problem.
Amazon S3
The main reasons to use Amazon S3 are that it's amazingly scalable and incrediably cheap. Other than that it's pretty easy to manage using the plugins or some gems around there, and it's generally offloading the weight of sending files to your users an keeping your application servers busy (i wrote before on apache's x-sendfile header, that's another way).
and... still.. BLOBs
As i said before, i am not a fan of storing files in your db, seems like out of context for me, but some people like it.
If you insist (and you shouldn't) , you'll need to setup a proper migration to handle BLOBs, and the rest is up to you.
Live Free: Open Source Software indexes
- OS Living - Great index, cut into categories.
- Wikipedia - List of free open source software.
- OpenSourceList - Anthoer category based list, some of the stuff here are Close Source, but still free to use.
- 50 Apps to manage your Office with open source - This list consists over linux based programs, but i think that Ubunut is anyway the number #1 replacement for windows in offices (except mine where everyone got a mac, haha).
- 5 Business free applications - why pink?! :)
Debugging Javascript in Web Applications: Major browser roundup

Javascript is an exception, it's a de-facto web development script language, but it's rendering engine and debugging options vary from browser to browser.
I gathered up a list of common web debugging tools for Javascript. i will be happy to get some more ideas from everyone.
here we go:
The ultimate solution - Firebug addon
ok, i know what you might say: "it's a FIREFOX addon!, how can it be THE ultimate solution?".
Well, good news.
Along side the plain firefox addon, there's also a lite version that runs as a seperate javascript on Safari, Opera and yes, IE.
The Lite version is not as comprehensive as the addon, but it's more than a blessing when dealing with IE javascript bugs ("line:33, char:33 crap").
General
JSLINT.COM - Javascript verifier
Just paste your JS code and let this cute little tool and watch the Javascript errors flow back at you. important to add that it does not recognize frameworks conventions (the jQuery $ selector is considered as a Global definition, use JQuery instead) and will not identify browser specific issues, it's marely a javascript syntax checker.
BlackBird.js - Cross browser javascript logger
Blackbird offers a dead-simple way to log messages in JavaScript and an attractive console to view and filter them.
Internet Explorer - Evil, annoying, can't we just drop it?
DebugBar - Firebug's little brother
debugbar is a nice Firebug mockup for IE, simple but not as strong as Firebug. problems are that it's not free, and you'll have to install a whole lot of crap on your computer. too bad.
you can also checkout the Compainion.js free version of a javascript console for IE.
IE Developer Toolbar - HTML, CSS, no Javascript
Let's face it, the IE's so-called javascript engine just sucks, any attempt they'll do to fix it must start by simply re-fuckin-write it.
The IE Developer Toolbar is a poor attempt to find a solution for some of the problems facing a web developer when dealing with IE, but at least it's something.
Safari - When i am not working, that's the one.
*by saying Safari, i mean the MacOS one, not that fucked up Windows version, yak.
Safari came from Apple, so it's probably padded with thought, intention and considiration to any kind of end-user, as well as a developer.
The Safari developer tools are nice, from a very descriptive, good looking Console and Network stats, to a rendering engine that can mimic other browsers (although i am not sure how good it is.).
This "How to Debug Javascript in Safari" article contains all you'll need to know.
FireFox - by the developers, for the developers
I have to say it, but if you made it this far and you still havn't figured out how to debug Javascript in Firefox, you have a problem.
Debugging Javascript is all about the Addons engine. This huge gallery contains a lot of web development related addons and tools, just go there and pick what you want.
Two addons you'll have to pay attention to are the Web Developer toolbar addon, and Firebug we've mentioned before.

140 Characters Web applications
I guess it right, you too i guess. "Twitter".

Rails and Facebook: Why (still) iFrame is better than FBML
What most people don't realize, is that there is not need to choose.
You can (not easily though) to get your iFrame application to handle and use FBML tags in a cool hybridy-ee way, and by that scoring the most points you can with both approaches.
Using iFrame has it's issues of course, you are going to lose primeraly the basics of the Facebook UI tools (tabs, borders and such) and you'll find yourself sometimes having rendered the entire Facebook layout in your iFrame, thau causing it to appear twice (some say twice too many).
What's good about choosing the iFrame approach is that:
- Complete control over your content. You’re essentially communicating back and forth with your own server; no middleman. FBML requires that you return your content, which can contain html and fbml, back to Facebook. They parse it and render your content.
- Javascript/Ajax considerations. FBML application type parses all your css and javascript and prepend all styles and methods with a unique app number such that all methods go from ‘do_this()’ to something like, ‘app_23423423_do_this()’. This creates a lot of extra work for you, especially if you are using Ajax in your app. Further more, some events are not allowed at all, while listeners are allowed.
- iframe allows you to develop locally.
- Rails and RESTful routing. If your application takes advantage or RESTful routing and you choose FBML you will be required to adjust for the fact that all requests to your callback url from Facebook are POST requests, which obviously is a pretty big problem.
There are some things you don’t get, but they are mainly UI things like the styles and tabs that make your content look more like Facebook content. Even if you choose iframe, you can pass the param
fb_force_mode=fbml
to take advantage of the things that really are useful such as the request forms and other FBML tags (therefore the hybrid theory :) )

Rails Facebook App Enlightments: HTTP timeouts
Since i had to handle so much crap with Facebook, Rails and the Facebooker plugin in order to get my latest application up, i decided to share a little bit with other innocent coders straying in the path of hell.
The Facebooker plugin interfaces with the Facebook API using the Net::HTTP ruby library and POSTs API requests (API call, FQL..) using the #post method (eventually, there's a whole bunch of stuff happening before that).
It sounds nice, it really does, problem is that in this specific app we have send a whole bunch of such requests to facebook, some requests return 1000-17,000+ strings back as JSON.... what makes Facebook simply.. stale.
Now getting like 3,400 Timeout exceptions raised in your production servers (adding that the CPU is on 3.2%, RAM is at 10%) is annoying. really annoying.
The "patch" we found for this issue was to ensure a specific amount of retries to be made by rescuing the Timeout Exception.
def get_my_somefing_from_fb retry_count = 0 begin do facebook crap rescue TimeoutError if (retry_count < 5) retry_count+=1 retry end end end
Now we are watching it closely to see exactly how it helps us, if at all.
Twiters Around
About Me
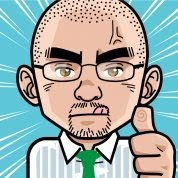
- Elad Meidar
- I am a web developer for more than 9 years, managed, cried, coded, designed and made money in this industry. now trying to do it again.
Blog Archive
-
▼
2009 (67)
-
▼
March (9)
- Moved to Mephisto
- Rails Nested Resources Tutorial
- Raphael: Amazing vector graphics Javascript Libraty
- Storing Files in Ruby on Rails
- Live Free: Open Source Software indexes
- Debugging Javascript in Web Applications: Major br...
- 140 Characters Web applications
- Rails and Facebook: Why (still) iFrame is better t...
- Rails Facebook App Enlightments: HTTP timeouts
-
▼
March (9)
Labels
- 1.9 (1)
- 2.0 (1)
- 2.2 (1)
- 2.3 (1)
- 2.x (1)
- accessibility (2)
- account (1)
- actionmailer (1)
- activerecord (3)
- adsense (1)
- affiliate (1)
- ajax (3)
- amazon (4)
- analytics (1)
- api (3)
- application (1)
- array (1)
- associations (1)
- attachment_fu (1)
- autocomplete (1)
- aws (2)
- blog (4)
- books (4)
- boolean (1)
- browser (8)
- browsers (3)
- bugs (4)
- buzz (1)
- callback (1)
- callbacks (1)
- caller (1)
- capistrano (1)
- chronic (1)
- class (2)
- classes (1)
- classifieds (1)
- client (1)
- coding (2)
- collboration (1)
- console (2)
- convert (1)
- core (2)
- core. object (1)
- cost (1)
- css (7)
- database (10)
- date (3)
- dating (1)
- db (2)
- debug (2)
- deploy (5)
- deployment (3)
- design (11)
- development (5)
- dojo (1)
- eager (1)
- ec2 (2)
- effects (1)
- elad (2)
- email (3)
- engine (1)
- engineyard (1)
- english (1)
- entrepreneur (1)
- environment (2)
- erd (1)
- error (1)
- example (1)
- expire (1)
- explorer (1)
- extention (1)
- extra (2)
- facebook (2)
- fast (1)
- file (1)
- files (1)
- firefox (4)
- fixtures (1)
- font (1)
- form (1)
- framework (16)
- free (6)
- funny (2)
- gem (7)
- gems (2)
- git (5)
- globalize (2)
- gmail (3)
- google (8)
- graphes (2)
- graphics (1)
- guides (4)
- hacks (1)
- hosting (1)
- html (1)
- http (1)
- ie (5)
- ie8 (1)
- image (2)
- indomite (1)
- install (1)
- iphone (1)
- israel (2)
- javascript (19)
- JQuery (10)
- js (3)
- legacy (1)
- leopard (1)
- lib (1)
- library (3)
- links (2)
- list (1)
- loading (1)
- log (1)
- logger (2)
- logging (1)
- love (1)
- mac (4)
- mail (2)
- manuals (1)
- mapping (1)
- mass (1)
- mephisto (3)
- mercurial (1)
- meta (1)
- metaprogramming (3)
- microsoft (1)
- migrations (2)
- minimagick (1)
- mistake (1)
- mistakes (1)
- model (1)
- money (4)
- mootools (4)
- move (1)
- movies (2)
- music (1)
- mvc (2)
- mysql (2)
- native (1)
- nested (1)
- network (1)
- new (1)
- newsletter (1)
- num_to_english (1)
- numbers (2)
- object (1)
- observer (1)
- ohad (1)
- open (1)
- operators (1)
- optimization (4)
- oracle (4)
- overload (1)
- parse (1)
- pdf (4)
- php (3)
- plugin (11)
- plugins (7)
- programming (4)
- programs (1)
- projects (5)
- prototype (1)
- queries (1)
- rails (50)
- rails 2.0 (10)
- rails 2.1 (6)
- rake (2)
- rants (1)
- record (1)
- reflections (1)
- release (3)
- rendering (1)
- reset (1)
- resize (1)
- rest (1)
- rjs (1)
- ror (1)
- routes (3)
- routes.rb (2)
- ruby (49)
- ruby on bells (2)
- ruby on rails (28)
- ruby-debug (1)
- s3 (2)
- salary (1)
- scaling (2)
- schema (2)
- screencasts (1)
- script (4)
- scripts (1)
- search engine optimization (1)
- security (3)
- seeking alpha (1)
- select (1)
- sendmail (1)
- seo (2)
- sequencer (1)
- sessions (1)
- share (2)
- shell (1)
- site (3)
- site map (1)
- sites (1)
- skype (1)
- social (2)
- source (1)
- source control (4)
- sprites (1)
- sql (1)
- stand alone (1)
- standards (1)
- startup (6)
- storage (2)
- subversion (4)
- svn (1)
- team (1)
- technology (1)
- testing (1)
- textmate (1)
- thread (1)
- time (1)
- tips (45)
- tools (5)
- tracking (1)
- tricks (36)
- tutorials (5)
- ui (4)
- uml (1)
- update (1)
- upgrading (2)
- url (1)
- usability (2)
- user interface (7)
- validations (1)
- vector (1)
- vote (1)
- web (9)
- web services (2)
- web2.0 (4)
- webistrano (1)
- wire (1)
- wwr (1)
- xhtml (1)
- yahoo (1)
- yui (1)
- רובי (8)
- רובי און ריילס (7)