Yaiii!
finally.
If you are here it means that you have an old link, or got redirected by a old RSS entry, please refer to my new blog "Emphasized insanity" and update your RSS subscription.
Ruby On Rails and a Conning Israeli entrepreneur
Moved to Mephisto
Rails Nested Resources Tutorial
Preface
The best way to get started with Rails 2.x nested routing and routing at all, is to read the official Rails Routing guide at the Rails Guides website.Rails Nested Routes/Resources
The rails nested routes/resources were indroduced at Rails 1.2 as part of the RESTful approach that was adopted by the Rails core members.Nested resources allowed urls like:
/tasks/?project_id=1
To have a bit more sexy/RESTful look:
/projects/1/tasks
Let’s use this example to make things a little more clear, we will use 2 models, Project and Task
# /app/models/project.rb
class Project < ActiveRecord::Base
has_many :tasks
end
# /app/models/task.rb
class Task < ActiveRecord::Base
belongs_to :project
end
Setting up routes.rb
Creating a Nested Resource
:has_many keyword
The easiest way to create a nested route, is to use the :has_many keyword like that:# /config/routes.rb
map.resources :projects, :has_many => :tasks
# and the correspondent task resource
map.resources :tasks
Adding the second routes, that defines a RESTful route to :tasks, depends if you would like to allow an access to the Task resource, without the project context, this is not a must.
Block
You can also specify the sub-resources in a blockmap.resources :projects do |project|
project.resources :tasks
end
Singular Resources
Just the same:map.resources :projects do |project|
user.resource :design_document
end
Routes Helpers
Run:$ rake routes
to see what kind of routes do you have in your application, you can pipe UNIX’s grep command (”| grep xxx”) to filter the results:
$ rake routes | grep project
A basic map.resources :projects will produce:
events GET /projects {:controller=>"projects", :action=>"index"}
formatted_projects GET /projects.:format {:controller=>"projects", :action=>"index"}
POST /projects {:controller=>"projects", :action=>"create"}
POST /projects.:format {:controller=>"projects", :action=>"create"}
new_project GET /projects/new {:controller=>"projects", :action=>"new"}
formatted_new_project GET /projects/new.:format {:controller=>"projects", :action=>"new"}
but a Nested route, like we defined before will produce:
project_tasks GET /projects/:project_id/tasks
new_project_task GET /projects/:project_id/tasks/new
edit_project_task GET /projects/:project_id/tasks/:id/edit
project_task GET /projects/:project_id/tasks/:id
very nice.
Singular Nested Route Helpers
(from the example above)
/projects - list all projects
/projects/1 - show a single project
/projects/1/design_document - a project’s design document
Using the routing helpers
Since we now have another resource in context when we want o use the new helpers, we need to include that resource instance as a paramter:
new_project_task(@project)
# or when both resources are required
edit_project_task(@project, @task)
Forms
I'll assume you use form_for in your forms, it will make the usage of nested resources a lot easier than to work with plain HTML or form_tag.
The regular form we know of form_for, receives one instance as the form object:
<% form_for(@project) do |f| %>
...
<% end %>
But with nested resources, we'll pimp it up a little bit:
<% form_for([ @project, @task ]) do |f| %>
...
<% end %>
Note the instances array, that specifies the objects we need in our form when we deal with nested resources
Conclusion and some Gotchas.
Using nested resources and routes is the right thing, URLs are clear, and code is readable. but:
# You should not implement nested resources of more than 2 levels.
# Setting up pagination support (?page=3) kind of breaks the RESTful approach.
# The railscast about Nested Resources.
# Using RESTful ajax calls, a great lib by dfr|work (#rubyonrails).
Raphael: Amazing vector graphics Javascript Libraty
Raphaël is a small JavaScript library that should simplify your work with vector graphics on the web. If you want to create your own specific chart or image crop and rotate widget, for example, you can achieve it simply and easily with this library.
Raphael Supports Firefox 3.0+, Safari 3.0+, Opera 9.5+ and Internet Explorer 6.0+ which is pretty awesome and allows you to do almost any graphic task you have in mind, just check the demos.
Raphael weighs about 110kb uncompressed, which is kind of an issue i think.
Get it here at the Raphael Homepage
Storing Files in Ruby on Rails
I finished my post before reading theirs and didn't add things Koz wrote and i didn't, so don't blame me in stealing or something. :)
I Know storing files in a database is usually a bad idea, but i still thought about the face that i was yet to see a propar guidance on how to handle files (using the correct practices of course).
Plugins
Attachement_fu - The older brother, pretty nice, better than the late file_column.
PaperClip - An "updated" attachement_fu, some people say it's better than attachement_fu because it is actually working. There is a lot more work being done on this plugin now a days, so it's better to relay on it rather than on attachment_fu.
Storing your files.
Local File System
This option can only work out for you, if you are running your app on a single server, simply set one of the plugins to store the uploaded files whereever you want, and that's it.
You can also setup a network folder if you'd like and imitate a local folder across a number of servers, but i's the same.
What you do need to be careful from, is a situation where you are saving too many files into a single folder, which will cause mainly for a slow file lookup.
Try to refine you directory structures for your upload root in order to avoid such a problem.
Amazon S3
The main reasons to use Amazon S3 are that it's amazingly scalable and incrediably cheap. Other than that it's pretty easy to manage using the plugins or some gems around there, and it's generally offloading the weight of sending files to your users an keeping your application servers busy (i wrote before on apache's x-sendfile header, that's another way).
and... still.. BLOBs
As i said before, i am not a fan of storing files in your db, seems like out of context for me, but some people like it.
If you insist (and you shouldn't) , you'll need to setup a proper migration to handle BLOBs, and the rest is up to you.
Live Free: Open Source Software indexes
- OS Living - Great index, cut into categories.
- Wikipedia - List of free open source software.
- OpenSourceList - Anthoer category based list, some of the stuff here are Close Source, but still free to use.
- 50 Apps to manage your Office with open source - This list consists over linux based programs, but i think that Ubunut is anyway the number #1 replacement for windows in offices (except mine where everyone got a mac, haha).
- 5 Business free applications - why pink?! :)
Debugging Javascript in Web Applications: Major browser roundup

Javascript is an exception, it's a de-facto web development script language, but it's rendering engine and debugging options vary from browser to browser.
I gathered up a list of common web debugging tools for Javascript. i will be happy to get some more ideas from everyone.
here we go:
The ultimate solution - Firebug addon
ok, i know what you might say: "it's a FIREFOX addon!, how can it be THE ultimate solution?".
Well, good news.
Along side the plain firefox addon, there's also a lite version that runs as a seperate javascript on Safari, Opera and yes, IE.
The Lite version is not as comprehensive as the addon, but it's more than a blessing when dealing with IE javascript bugs ("line:33, char:33 crap").
General
JSLINT.COM - Javascript verifier
Just paste your JS code and let this cute little tool and watch the Javascript errors flow back at you. important to add that it does not recognize frameworks conventions (the jQuery $ selector is considered as a Global definition, use JQuery instead) and will not identify browser specific issues, it's marely a javascript syntax checker.
BlackBird.js - Cross browser javascript logger
Blackbird offers a dead-simple way to log messages in JavaScript and an attractive console to view and filter them.
Internet Explorer - Evil, annoying, can't we just drop it?
DebugBar - Firebug's little brother
debugbar is a nice Firebug mockup for IE, simple but not as strong as Firebug. problems are that it's not free, and you'll have to install a whole lot of crap on your computer. too bad.
you can also checkout the Compainion.js free version of a javascript console for IE.
IE Developer Toolbar - HTML, CSS, no Javascript
Let's face it, the IE's so-called javascript engine just sucks, any attempt they'll do to fix it must start by simply re-fuckin-write it.
The IE Developer Toolbar is a poor attempt to find a solution for some of the problems facing a web developer when dealing with IE, but at least it's something.
Safari - When i am not working, that's the one.
*by saying Safari, i mean the MacOS one, not that fucked up Windows version, yak.
Safari came from Apple, so it's probably padded with thought, intention and considiration to any kind of end-user, as well as a developer.
The Safari developer tools are nice, from a very descriptive, good looking Console and Network stats, to a rendering engine that can mimic other browsers (although i am not sure how good it is.).
This "How to Debug Javascript in Safari" article contains all you'll need to know.
FireFox - by the developers, for the developers
I have to say it, but if you made it this far and you still havn't figured out how to debug Javascript in Firefox, you have a problem.
Debugging Javascript is all about the Addons engine. This huge gallery contains a lot of web development related addons and tools, just go there and pick what you want.
Two addons you'll have to pay attention to are the Web Developer toolbar addon, and Firebug we've mentioned before.

140 Characters Web applications
I guess it right, you too i guess. "Twitter".

Rails and Facebook: Why (still) iFrame is better than FBML
What most people don't realize, is that there is not need to choose.
You can (not easily though) to get your iFrame application to handle and use FBML tags in a cool hybridy-ee way, and by that scoring the most points you can with both approaches.
Using iFrame has it's issues of course, you are going to lose primeraly the basics of the Facebook UI tools (tabs, borders and such) and you'll find yourself sometimes having rendered the entire Facebook layout in your iFrame, thau causing it to appear twice (some say twice too many).
What's good about choosing the iFrame approach is that:
- Complete control over your content. You’re essentially communicating back and forth with your own server; no middleman. FBML requires that you return your content, which can contain html and fbml, back to Facebook. They parse it and render your content.
- Javascript/Ajax considerations. FBML application type parses all your css and javascript and prepend all styles and methods with a unique app number such that all methods go from ‘do_this()’ to something like, ‘app_23423423_do_this()’. This creates a lot of extra work for you, especially if you are using Ajax in your app. Further more, some events are not allowed at all, while listeners are allowed.
- iframe allows you to develop locally.
- Rails and RESTful routing. If your application takes advantage or RESTful routing and you choose FBML you will be required to adjust for the fact that all requests to your callback url from Facebook are POST requests, which obviously is a pretty big problem.
There are some things you don’t get, but they are mainly UI things like the styles and tabs that make your content look more like Facebook content. Even if you choose iframe, you can pass the param
fb_force_mode=fbml
to take advantage of the things that really are useful such as the request forms and other FBML tags (therefore the hybrid theory :) )

Rails Facebook App Enlightments: HTTP timeouts
Since i had to handle so much crap with Facebook, Rails and the Facebooker plugin in order to get my latest application up, i decided to share a little bit with other innocent coders straying in the path of hell.
The Facebooker plugin interfaces with the Facebook API using the Net::HTTP ruby library and POSTs API requests (API call, FQL..) using the #post method (eventually, there's a whole bunch of stuff happening before that).
It sounds nice, it really does, problem is that in this specific app we have send a whole bunch of such requests to facebook, some requests return 1000-17,000+ strings back as JSON.... what makes Facebook simply.. stale.
Now getting like 3,400 Timeout exceptions raised in your production servers (adding that the CPU is on 3.2%, RAM is at 10%) is annoying. really annoying.
The "patch" we found for this issue was to ensure a specific amount of retries to be made by rescuing the Timeout Exception.
def get_my_somefing_from_fb retry_count = 0 begin do facebook crap rescue TimeoutError if (retry_count < 5) retry_count+=1 retry end end end
Now we are watching it closely to see exactly how it helps us, if at all.
Rant: Why i Hate Facebook (API)
Through the past few weeks, I've been working on a facebook application, one of this year's todo list items, with my friend Lior Levin.
Well, it's been hell.
Not because of Lior :), but becasue of Facebook's horrible horrible API, i haven't seen such a bad API implementation since working on a Tranzila Credit card gateway in Israel, two years ago.
The facebook api and the entire application-programming-guidelines are idiotic, they offer the support of iFrame applications.
"good, actually great" you think.. "at least i woun't have to use the crappy FBML mishmash thingie... and i can use JQuery!" - But you are wrong, although all of these things are true, using the iFrame interface you are not touching any FBML crap and can use any JS Framework you'd like, but the horrors... the horrors.
First of all, each applications must have a set of callbacks assigned in the application's setting page: callback for application main gate, post authorization, post remove and another thing that doesn't matter now.
No RESTful web service support what so ever, except for the main application page, all other callbacks are POSTs, what's the problem?
When being a loyal Rails developer, you'd use RESTful routes almost by default, it's just right, but what do you do when Facebook decides that user removal actions should be POSTed and not DELETEd? you fuck up your routes.rb to match that, great.
The iFrame is a whole different story, if a user is yet to add your application, he will be redirected to an "Allow application to do this and that" page, what's the problem? that the redirection happend WITHIN THE IFRAME! which basically now causes the user to see a double facebook top bar from that moment until he refreshes the entire screen.
Crap.
It took me a while but i found a way passed it by flagging the first time a user comes in, and JS redirect the top document to an hard coded application url.
In this whole mess there was a single light in the end of the tunnel, which is the Facebooker plugin, which work great but it's documentation and online tutorial basically suck.
Maybe i'll do a new one...
done.
Startup Thoughts: Realty and a Dream
Everyone wants to be a big bad rock star entrepreneur, wake up to that morning when your bank account is flooded by plain cold cash, you are making millions while sitting at home and watching the download meter of your software or user count of your web site flying sky high every day.
Well, it's possible, many people have done that, and the nice thing about the web/internet world, is that there will be always, always, enough to share.
But it's a long way, and as the nature of things that take long, they tend to break our spirit, get us bored and insecure and afraid of competition.
I have gathered around a few misconceptions about startups and entrepreneurship, misconceptions that most people around this business seems to posses, but those who won't, these are the ones who break forward.
Misconception #1 - "Fast, Fast, Fast"
Well, shortly.. NO!
Nothing is more important than keeping your startup organized, Clear and planned ahead. Programming fast does not guarantee a better product, Designing, looking around a bit and gathering the baseline on which your consist your product, does guarantee a better product.
Get yourself a clear milestone plan, point out Alpha version (Standalone POC), private beta (for users you self pick) and a public beta, and stick with this plan!.
Misconception #2 - "We'll grow with it"
Scaling... people seem to forget about it, more like avoid it until the "twitter/dzone/digg affect" hit's their home page.
Web application tend to collect more users in a period of time than a downloaded software, web applications are also a single point of service (in case of SaaS), so you'd better get your system infrastructure ready and available for a user boost one day or another, viral marketing can be a dangerous thing.
Misconception #3 - "I've had enough"
i've heard people say "if this project doesn't go public in 5 months, i am out" or "i can't work with this people, i'm out". There's a word for people like that.
quitters.
and quitters don't get rich.
The people you work with may be annoying as hell, stupid as hell or useless as hell, if you truly believe in the concept of the idea, don't let anyone else's negligence to take what is yours and do everything you can (and you ALWAYS can) to get the startup up and running.
Misconception #4 - "We need more money"
Sometimes you do, sometimes you don't.
In order to get a website up and running these days, ready and eager to handle thousands of users, you only need AWS, which if you exaggerate like hell with your configuration, you'll get to 1000$ a month.
So following my previous post, you should know that one or more of your partners must be able to supply you with technical solutions (system administration, coding) so this should almost never be counted as an expense.
Most of the most successful startups were not founded, ever. keep that in mind.
Startup Thoughts: Sharing the cake
I've been involved in several startup projects lately. I the majority of them, one of the main reasons why founders and equity owners start to fight one another, is the moment in which someone thinks some equity needs to be shared with someone else, out side the current equity holders circle.
Well, nobody likes to give out equity and it's hard to know when and tho whom such company/project holdings should be granted.
The option of giving out equity, is mostly driven by the fact the founders need money to make their idea come to life, money or an equivalent needed resource (advisers, tech people). When there is no money, you need to ask yourself one question.
Do i REAALLLY need it?
.
They say that holding 100% of 0, is 0.. and they are right, if you will hold your equity holdings close to the chest and refuse to share no matter what, you'll probably heading straight down the hill. But on the other hand, giving out equity for anyone who wants some, or giving unreasonable amounts for that matter, is same as bad.
You can't do anything by yourself, you have to share your idea with some other people (unless you are really something special).
The first time you share your project equity with the other founders is simple and easy, most of the time the equity will be divided equally (and it doesn't matter who brought the idea, it's irrelevant) among the founders, stated in a partnership agreement later to be replaced by and incorporated entity legal documents.
Now when it gets to expanding the circle, there are two kinds of entrepreneurs that i can across with:
- Hard - These guys are either possessed with a too-high-self-confidence and sure that they can make it to the finish line without sharing a 0.1% with anyone else except for the initial founder circle or people with a wise project-wide view of things and insists of not giving away equity until it's absolutely necessary. He believe that any problem can be solved either by finding other forms of getting the required service/resource and will do whatever necessary in order to make things work out without having to release equity or unnecessary funds.
It's hard to distinct between these two sides of the Hard Core entrepreneur, but if you have the second kind of person in your team, you are a winner. - Soft - The soft entrepreneur is the the person who will be willing to give away anything for a little help. They will prefer to give away equity as much as possible like hot buns when it's not necessary and not required.
- "Don't care" - people who prefer not to take a stand, and will leave everything in the hands of other founder or all the others together. While this can be very useful when he goes your way, it's a problem when he's not.
I don't believe in workplace democracy, not all the time. Sharing equity should be unanimously passed if it is taken in a vote matter, or by a single responsible position holder (CEO for ex.).
Each one of the initial founders holds his share for a reason, he earned by contibuting to the success of the project and therefore should not be downsized unless he agrees.
You are not giving out equity to whoever comes your path, this list of people you should consider giving equaty to in exchage to their services.
- External CEO - You know how to program and design, but you don't know nothing about the business world and need someone else to guide your project to success. An external CEO is a good solution for that as long as he really understands what your idea is all about, what rarely happen.
Nobody knows your product better than you or your fellow founders for my opinion, but if you need a CEO, grant out about 10-12%. - Venture Capital Firm - The start-up Messiah, will generally have their own demands but it will move around 20%-60%.
- Strategic Investment - Someone who has the capabilities to bring your product to your desired market, audience and exposure. Depends on what he can give you, the appropriate share for such a service is 13-17%.
- Angel - A small financial investment that it's entire purpose is to get things running. 10%-15% is enough, but note that if it's a first financial investment, it's also sets your company value. (10% for 25K, brings your company to a 250,000 value, and your share grows as well.
- Tech Services - If you are going for a tech related project, such as a website or a software, make sure the other founders can supply as much as possible from the project's technical requirements, if you didn't (bad) and still need these services, grant about 0.2% for a programmer and 3%-4% for a team leader.
Keep in mind that anything that can wait, should wait and the Internet is a pretty big place for you to find what this people will probably charge 100$ an hour to tell you.
When you do need a lawyer or counseling, pay the money and that's it, no equity for counseling.
Last thing, keep in mind that there are wolves out there, people who seek to only find young, naive entrepreneur to which they can sell any dreams they want.
Consider every offer, but make the most to avoid sharing if you can, and if you do, share as little as possible.
The Lazy Web Developer: web 2.0 generators list
1. Web 2.0 Generator
It's really stupid, but you can't exclude a "Web 2.0 generator" named tool from a list with the same name.
Stripe Generator 2.0
Stir
Are you a fan of Tabs? Here is a handy web generator for you. With these tab generator, you can see what the page you will look like and when you are done creating just click download and it will give you the PNG file. That easy.
Button Generator
Well you got the Layout, badges, tabs What else can do need? Some buttons to offer the free downloads or maybe something else. Buttanator will help you with that easily generate web 2.0 . There are more button generators. I will add the link at the end of the post.
“A favicon (short for favorites icon), also known as a website icon, shortcut icon, url icon, or bookmark icon is a 16×16 pixel square icon associated with a particular website or webpage. (source)”
If you want to create professional a web site a favicon is a must. I have seen many websites that is a professional , nicely designed site but lacks a favicon. With these generator you can see the favicon in your browser in real time. If you have an image you can generate favcion from the image too.
Stop wasting your time on creating pre loaders. Instead use this generator. I absolutely love this site. They have all kinds of loader and you change the color and background. Also, this site has nice design as well.
Some of the web sites sometime gives out free vectors, photshop brushes and more. They put the images of the brushed on a realistic 3d box. Take a look at Bittbox example. It can be done in Photoshop or a good image editor. But why not use something easy. Just upload 3 images for the box (front,sides and top) in less than a minute you will have a 3d box with any kind format you want to download. Take a look at the box i created just using a random image. Isn’ t that great or what?!!!!!
If you are a photograppher or someone who has lots images on your site and make sure people do not steal your images, use this tools to watermark images. The cool thing about Picmarker you can watermark your images from your computer, facebook, flickr, Picasa album. The watermark again can be done using any image editors. But this tools allows you to watermark couple images easily.
Easily Create Rounded CSS corners with HTML and CSS.
Also check out…
- BGPatterns : Another useful patterns creator with symbols. Really useful site.
- Fresh Generator : Generate Web 2.0 buttons, rounded corners, boxes and more.
- Website Ribbon Generator: Another awesome generator. Nice, easy and beautiful.
- Free Post it Note Generator: Can this get any better? Generate Cool hand written Post it note.
- XHTML/CSS Markup Generator : If your are like me who forgets all the tags, use this tools to generate the markup.
- Reflection Maker: If you have an image you want to create a reflection, upload and choose the size and Your done! So easy and convenient.
- Genfavicon: Another Favicon generator.
- Web 2.0 logo creator: Create web 2.0 logos easily.
- Stripe Designer: Generate stripes with many different options.
- Tartan Generator: Forget stripes use tartan. This utility will help you to generate a nice tartan.
- Free Footer Generator: FreeFooter is an easy and fast tool that simplifies how you personalize your web site blog page footer.
- Fresh Badge : Anoter Brush Generator.
- Cool Text : Free Graphics and Button Generator.
- Color Scheme Generator: The Color Wizard is a color matching application for anyone who wants to create designs with great looking colors.
- WebFX: A web Based graphic effect generator. Choose an image from your site and let WebFX do the rest.
- Dotetemplate: Build and customize a whole website with this web generator.
- Avater Generator : Generate Web 2.0 avatar with text or more.
- Wordpress Plugin Generator: No idea! Check it out if t works and let me know.
- CSS sticky footer: Not a generator , a CSS technique to make your footer stick at the bottom of the page.

How to avoid screwing up a software project
Run Programmer, Run
When developing a new application, mostly when it's being done personally and alone, can sometimes be a very complicated process. you'll need to handle a whole bunch of other stuff than just coding (marketing, server setups even investors hunt) and it can sometimes lead you to a software neglection.Don't let it happen, run/compile your application every few days, keep it living in your head.
Sometimes you'll start work on some big shiny feature (e.g. adding a kewl Google maps integration), but stop because you hit a technical bump ("What? no maps for Israel?"), or don’t have the time to finish it ("Need to finish this Company profile by tomorrow") and The source code is left in unfinished state.
You can’t do anything with any of your code until this is fixed, and the longer you leave it, the more you’ll forget and the harder it will be to get started again.
This is called a "broken build", and is a big landmine because it impacts other peoples ability to work (And your ability to continue as well).
TIP #1: You started something? finish it before moving on to something else.
TIP #2: Stay in touch with your software.
"I Sure could use a time machine right now...."
Know these times when you wished you had a time machine? well, it can happen in the process of developing and application too.People make mistakes, Always. When people make mistakes in the kitchen, the food comes out really bad and you call the local pizza delivery services and solve the problem. When a programmer makes a mistake or is doing a system wide change... you'll need a ready to use Plan B around.
Source Control is the software world equivalent of a time machine, you can go back to a certain version of your application and rollback any changes made to your code and application, and by that, maybe reversing a very serious threat to your code.
If you haven’t taken the plunge with revision control yet, I highly recommend looking at some of the free SVN or GIT hosting services post.
TIP #3: Save yourself, use a source control service.
"Look at this cooooooolll JQuery accordation!"
Features are fun, Javascript magic is amazing, but you'll need to focus on what's really importantFocusing on things like validation, cool eye candies or extra functions is a great way to build up a large complex code base that doesn’t do anything useful yet.
Focus on the core functionality of your software first — your main features should be complete before you start thinking about WOW stuff.
Wasting trying to think of the perfect name, designing a logo or an icon, choosing the perfect open-source license and making a website won’t get you any closer to having a working application.
TIP #4: Core functionality first, fun - later.
Throw your code away and start from scratch - The Netscape mistake
As Netscape famously discovered a few years ago, throwing away existing code to start afresh is almost never a good idea. Resist the urge and make a series of small, manageable code re-factoring instead.TIP #5: Never sink your own boat, pickup a bucket and start pulling out water
Pull yourself together before you sit down
"mmm, Rails? or maybe Adobe Air? or maybe... maybe we'll do the cool gears thing?" Before doing anything ask yourself a simple question - "What are you actually trying to achieve?", Spend some time with a pen and some paper coming up with a really clear vision of what you’re trying to create — e.g. screen mock-ups, basic core functionality (yes, again) and if there is a process you are trying to imitate, go through it too.
TIP #6: If you don’t know what you’re doing from the beginning, you’ll have no chance of finishing it.
Get the right men for the job
You maybe a coding genius, a super-programmer (Mike it's you) or other superior programming entity. but unless you are a really something no one had ever seen before, you don't know anything there is to know about every aspect of developing a new software and a products.If you can't tell the difference between red and green, get a designer to work for you. if you don't have a clue in CSS or DB infrastructure, get someone who know this art to do it.
TIP #7: Do what you know, not what you don't know.
Marketing is Important, but when it's time
Tell people about your product when you have something to show, open a product twitter account when you have something to say, don't rush yourself forward and try to stick the product or create a hype when you can't back it up by at least screenshots or an actual product on the best scenario.TIP #8: Invite people in when you have something to offer them to eat.
The difference between a lie and a promise
Users don't mind waiting for features, they mind finding out you promised them something and didn't deliver.Stand up to your words, and update your users about development progress and new features on the way, as long as you really intend to do it and not just saying to make them download or register.
TIP #9: Users are like your mother, lie to them, and they'll know.
Rails 2.3: Weird multipart form bug
Edge versions, such as the latest Rails 2.3 RC0 release often break a lot of core operations, and that's why it's called RC
Yesterday at #rubyonrails on freenode, some dude came by with weird weird bug regarding one of it's application's forms:
When he has 2 file upload columns, and a free text field between them, upon submitting the form the text area value is no where to be found on the params hash.
After trying to help him and try to approach this matter from several directions with no success, he came up with this ticket he found on the Rails code ticket report system - "Posting a multipart form with file data can break params assignment (in 2.3)".
My advice: wait a while before you use edge, unless you really intend of finiding these bugs, that's OK... just don't try that for production.

Update: Social Network Ruby on Rails Applications
Update 28/1/09 : Added El-Dorado
Update 27/1/09 : Added OneBody
Update 12/2/09: Added EchoWaves, OpenMind and Forulio
One of my current consulting positions, involves a startup company with a great idea and a great social network implementation idea.
For that matter, i have been scavenging the web lately for Rails based social netowrking plugins, Engines or maybe even a skeleton application for those guys and i found some very good solution apps and infrastructures, the only problem rails Rails 2.0 compatibility (which i didn't check yet, and some don't indicate) and Documentation, which seem to be neglected on almost all those apps.
So, Here's the current list:
LovdByLess
include blogs, user profiles, photo uploads, forums, friendships and user-to-user messaging funcionallity.
Looks great and has a great look and feel.
Comes as a full application under MIT license.
CommunityEngine
A Rails engine, now supports Rails 2.0.
Gives you the basic functionality for a social networking site. It includes features such as User pages, forums, uploads, geo searches, blogging and more.
Important to say that extending the basic engine with your own controllers and models, is supposed to be easy and simple, i am currently testing it out so i don't really have an opinion yet.
Documentation has to be improved though, a lot.
TOG, presentation
TOG is basically a package of features (in the form of plugins) which you can choose to add to your applications as one, or as a whole.
Using these prepackaged and automated features should let you focus on the real key point about social networks: keep the conversation going with your members. If you’re just starting a social site or wants to polish your community with lovely social capabilities maybe you should give tog a try.
Insoshi
Features include:
- Activity feed
- Personal profiles with photo upload and comment walls
- Internal messaging system with read/replied/trashed messages
- Contacts list
- Blogs with comments
- Discussion forum
- Search for profiles, forums, and blogs
- Admin panel with site preferences
- Option for email verification and activity notifications
LuvFoo
Under developement, but runs several sites at the moment,
include some neat features on top of the plain simple ones, such as API and CMS intergration.
OneBody
Although i am not sure why they indicate their audience as Churches (?), it looks like a good social framework with a lot of features such as:
- Membership Management | aka ChMS
- Online Directory | search, print
- Groups | email lists, attendance tracking
- Social Networking | friends, favorites
- Content Management System | page editing
El-Dorado
Looks nice and packed, a pretty live demo site too.
Features include:
- Forum
- Group chat
- Event calendar
- File sharing
- Random headers
- Avatars
- Themes
- Privacy settings
EchoWaves

This is a group chat social network application. You can start conversations and connect wih other users while discussing it.
It is possible to make a conversation read-only for presenting a content too.
Openmind

Openmind enables you to create a collaborative environment for collecting the thoughts of your customers/collagues on a product/service.
User can discuss online about an idea & vote for the features. Admin can mark the most-requested features as "to be included in next releases" & users get informed from this. A nice application for creating a constructive community.
Forulio

Forulio is a Ruby on Rails forum application with a similar approach to popular forum softwares like phpBB or vBulletin.
It supports sub-forums, has a monitoring page for viewing the activity and has tagging integrated.
Other resources:
- A Post at Hykoo.com about the hunt for a rails social networking solution.
- Agilewebdevelopment.com Plugins depot, Many plugins that may fit your social network needs and features.
- a book, Practical Rails Social Networking Sites (Expert’s Voice) : By Alan Bradburne: Supports Rails 2.0, worth every penny (we use it as a training guide for new developers).
- Great step by step guide to create a social network in rails up on MissingMethod blog, it's pretty while back so i suggest trying to find some recent plugin replacements though.
Mockups: Easily create application mockups
This Mockups tool, by Balsamiq, is one of the best tool that i had seen for this job.
It's an amazing tool, combining a beautiful interface of it's own, a vast library of UI elements and an overhaull feeling of "planning".
The only downside is of course the price, 79$ is more than Textmate's price tag... i can say that in a price of 50$, it's a must have.
Big thanks to Lior Levin for this tip.
FireFox blogging extention: ScribeFire
Iv'e tried several Mac clients (MarsEdit, Ecto) and none of them succeeded in working with my blog (probably a template issue, not resolved yet).
A few days ago i came across this Blogging firefox extention called ScribeFire, which appears to be the ultimate solution.
ScribeFire supports multiple blog platforms, HTML editing and even an embedded ads service (didn't try it though).
Rails Conditional Eager Loading
Rails's eager loading (ScreenCast) is an important practice that saves some requests and queries when dealing with table relations in rails.
Up until now, using a condition hash to specify query was a little tricky if you use the eager loading method:
User.find(:all, :include => :jobs, :conditions => ["jobs.salary > ?",1000])
or using a hash (without eager loading)
User.find(:all, :conditions => {:name => 'eizesus'})
Using a conditions array/hash isn’t always my favorite way to write finds in Rails, but in certain cases must have when you deal with complex queries (like and "Advanced Search" feature).
In my current work,I needed to run conditions on an associated table in just such an "Advanced search" feature, so i came up with this simple and easy way to use a condition hash for eager loading methods:
User.find(:all, :include => :jobs, :conditions => {:name => 'eizesus', :jobs => {:position => 'Manager'})
or you can prepare the condition hash on the fly:
conditions = {}
conditions[:first_name] = params[:first_name] unless params[:first_name].blank?
conditions[:last_name] = params[:last_name] unless params[:last_name].blank?
conditions[:jobs] = {:position => params[:position]} unless params[:position].blank?
@users = User.find(:all, :conditions => conditions, :include => :jobs)
2 Useful CSS tips
"Don't look for water when something burns, just don't play with fire" - My Mom.
Preventing bugs before they happen, that's the key.
If you don’t prevent bug, they will start a snow ball that will force you on and on with ridiculous patch-work and what i basically call the "fire extinguishing loop", there will be a lot of them interacting with each other, making it difficult to figure out what’s what, and were it all began from.
The majority of bugs reside in JS differences between browsers and CSS and DOM interpretation, so i'll try to specify some methods and techniques we use here at my company, who make our lives a lot more easier.
CSS Resets
asterisk CSS selector:* {margin: 0; padding: 0;}
But, it's too rough. Using this technique cause my forms to get messy and got me upset since i had to define every single padding and margin specification.
ince then, I’ve been using the popular CSS reset by Eric Meyer, which collects a set of such reset attributes, for several common HTML tags.
Of course i had to tweak it to fully match my needs, but still , it's a must-have baseline for cross browser CSS development.
Clearing floats
IE6 does not like floats too much, IE8RC1 is not a big fan either, especially when those floats are not clear.Uncleared floats are often the cause of major bugs. however, it’s a bug that is easy to fix.
Setting an HR element with width of %1 and setting it to clear:both, will solve the problem.
<div id="container">
<div class="floater">
floating.
</div>
<div class="floater">
floating.
</div>
<hr class="clear"/>
</div>
<span>I am below!!</span>
and the CSS:
#container{
}
.floater{float:left, width:60px; margin:6px; padding:6px; border:2px solid #888;}
hr.clear{clear:both;width:1%;}
ther clear:both definition orders the HR element to go down one line if the is any other element on it's left or on it's right, read more on the clear CSS attribute.
IE8 RC1: IE6 again?
IE6 was, and still is the worst nightmare for web developers (client side mostly).
This browser/disease who surfaced the Web years ago, still acts as a major market share holder (about 67% these days) and hurts users, developers and basically everyone who comes across it's path.
I am a web developer and there are many in my profession who can really understand this evil browser, much more than the common user who think the "internet is the blue icon...no?", we're still dealing with CSS Hacks, HTML and JavaScript that IE6 forced us to write, PNG manipulation (what did we ask for?! transparency?) and much more.
So the nearing release of IE8 should be good news, right? Everybody moves up a notch, even the poor people who have to use IE6 in the working place (yeah, there are more than a couple of these companies in Israel).
Not so fast. What made IE6 so bad is that it's bugs became standards. That people accepted it's negligence as a permanent status and began to work around it's bugs around the clock, forcing themselves and other to years of web-desperation.
On the other hand, these people web sites usually were their paychecks, so they really didn't have too much time to wait for dear old Microsoft to release a patch/fix/update that will fix their specific bug (and probably bringing 300 other bugs), so they hack CSS, HTML, JavaScript and Images in order to make it work. Before they knew it, the bugs and other demented issues from the IE standard demonic bugs, have become the standard.
After trying out IE8 RC1, i can say that i am worried, Tables that don't render correctly, JavaScript calls that crash the browser into oblivion and meta tags that order the browser to render the page as IE7, get real.
It's not just the bugs, it's the fact that the IE8 development seems to be giving these bugs the backend, it's been there, and have been reported already in Beta (i know i did).
If IE8 makes it out with this huge list of basic usage bugs, we are going on round 2 with IE6, And all of us web developers and designers will travel back in time to 2002.
IE6 for my opinion was the reason that almost nothing moved around web development and web applications between 2002/3 up until FireFox came along, when there was no competition, IE rules with it's bugs without nothing to worry about and with nobody has the ability to point out the exact things he wish to have in a browser, so Microsoft did nothing until FireFox became strong enough to threat the king.
Although that i would be happy to extinguisher the very existence of the IE family, i will be very happy to see a normal, advanced browser coming from Microsoft.
So, if you want to make sure that IE8 isn't a new IE6 in a new outfit, get yourself a copy of IE8 and start shooting some feedback at Microsoft. Also, vote up those bugs that really need fixing. Even if you don't use IE in your day-to-day work, if you develop web software, this is a matter of raising the bar, before they make a new one, very very low one.
Get Internet Explorer 8 and get to work.
CSS Frameworks Tutorials and getting started guides
First thing, it helps.
Exactly the same as in Server side languages and client side languages, frameworks are a great improvement (in most cases) to a regular off the board development.
There are a lot of CSS frameworks these days, Noupe guide to CSS framework gathers the best of the guides and tutorials for each of the major frameworks.
I personally tried to use several of them and i guess the 960 CSS framework proven to be the easiest to handle (for me, and i usually don't do CSS).
Ruby 1.9 is out
source Ruby Inside
Benchmarks say it's twice as fast as 1.8.7, but developers worn not to use it as a production ruby rght away becouse it may a lot of libraries and Gems.
so, be worned.
Amazon AWS: Calculate your monthly costs on Amazon S3, EC2 and SQS
"its' 0.3 cents per hour, this is 0.32 cents per request"
So how can we get the subtotal cost of an AWS Cloud application?
Simple, use the Amazon Cloud Services Cost Calculator.
note: i tried it with my own parameters, and it's showed a little above what i actually pay (5$ more).
Noupe: 15+ Incredibly Useful Mac Apps For Freelance Web Designers
Noupe: 15+ Incredibly Useful Mac Apps For Freelance Web Designers . <== This is a great blog, don't dare not to subscribe to their RSS Feed.
1. Espresso
Extremely powerful web development tool, created by the minds behind CSSEdit. Providing a powerful editing, sleek projects, live preview, real publishing and synchronization tools.
Espresso features an immensely powerful rule-based syntax engine, CoreSyntax, that transforms your text documents into semantic structure. Espresso has an extensible Navigator that’s best compared to the CSSEdit styles list.
2. CSSEdit
CSSEdit’s unique focus is on style sheets, it offers a wide range of features for any level of expertise. Starting out? Selector Builder, advanced visual editors, Live Preview, intelligent source environment and a powerful X-Ray web page inspector for an unbeatable CSS debugging suite.
3. Pixelmator
Pixelmator, the beautifully designed, easy-to-use, fast and powerful image editor for Mac OS X has everything you need to create, edit and enhance your images. Pixelmator is a layer-based image editor. You can quickly create layers from your photos, other pictures, from selections or even your iSight. Pixelmator can add a layer to your composition directly from your Mac’s little camera. With Pixelmator’s powerful, pixel-accurate collection of selection tools you can quickly and easily select any part of your images to edit and apply special effects to portions of your pictures.
4. Coda
Panic Coda is a great all-in-one Web development environment tool, keeping many of your commonly used tools in separate tabs. Tools include: text-editor, css editor, file transfer, svn, terminal & smart Spelling. All of the usual languages are supported and styled appropriately including: CSS, HTML, Javascript, Java, Perl, PHP, Python, Ruby, SQL, XML, and straight text.
5. Lineform
Lineform is an ideal Mac app for vector art, diagrams and illustrations. Lineform has all of the most popular tools, including everything from freeform gradients to compositing effects, enabling you to create the designs you want without getting in your way with superfluous “features” you don’t need.
6. Fontcase
Fontcase is a font management application that provides an elegant and powerful workflow to help you organise the fonts you have installed on your system. Designed to be an iTunes for your fonts, Fontcase has a powerful tagging system, which is designed to let you control your fonts like you control your music.
7. Deep- Image Search
Deep calculates the range of colors used in each of your images by analyzing the pixels and calculating the most popular colors. This is what makes the palettes in Deep powerful, you can find images that have similar colors, which is great when you are looking for the perfect picture to go on your web site. In fact, just drag any image on to Deep and it will find and rank all the images on your computer that are similar.
8. Cyberduck
Cyberduck is an open source FTP, SFTP, WebDAV, Mosso Cloud Files and Amazon S3 browser for the Mac. It features an easy to use interface with quickly accessible bookmarks.
9. Flow
Flow is another file transfer coming in style. Flow brings the best of the Mac to your server’s files and folders. Put simply, Flow makes working remotely every bit as intuitive and natural as working locally with the Finder. With Flow, you can edit files directly on your server, connect to (FTP, SFTP, Amazon S3, WebDAV, and MobileMe iDisk servers), Quickly Upload Without UI and many other features.
10. Sequel Pro
Sequel Pro is a Mac OS X MySQL Database Management app. Sequel Pro gives you direct access to your MySQL databases on local and remote servers with support for importing and exporting data from popular files including SQL, CSV and XML.
11. Things
Task management has never been this easy. Organize your to-dos with Projects and Areas of Responsibility. Features include: A smart Today list automatically gathers all you need to look at, Repeating To Dos, Every list can easily be filtered and sorted by due date, iPhone Sync and many other features.
12. xscope
xScope is a powerful set of tools that are ideal for measuring, aligning and inspecting on-screen graphics and layouts. Quickly available via the Mac OS X menu bar, xScope’s flexible tools float above desktop windows and UI elements making measuring a breeze.
13. KeyCue
KeyCue gives you an instant overview of the overall functionality of any application, plus lets you automatically start working more efficiently by making use of menu shortcuts.
14. TextExpander
TextExpander saves you countless keystrokes with customized abbreviations for your frequently-used text strings, code snippets and images.
15. OnTheJob
On The Job has a lot of power under the hood but maintains it’s reputation as the easiest to use time and expense tracking solution available for Mac OS X. On The Job offers flexible invoice creation. Create invoices for specific date ranges, covering a single job, or spanning multiple jobs.
Other Mac Apps you will find interesting
- TextMate
- Billings
- Littlesnapper
- MAMP
- Inkscape
- Picturesque
- Layers
UML for Rails applications
UML is a Graphical language for visualizing, specifying and constructing the artifacts of a software-intensive system. The Unified Modeling Language offers a standard way to write a system's blueprints, including conceptual components such as business processes and system functions as well as concrete things such as programming language statements, database schemas, and reusable software components.[1] UML combines the best practice from data modeling concepts such as entity relationship diagrams, business modeling (work flow), object modeling and component modeling. It can be used with all processes, throughout the software development life cycle, and across different implementation technologies.
As the strategic value of software increases for many companies, the industry looks for techniques to automate the production of software and to improve quality and reduce cost and time-to-market.
These techniques include component technology, visual programming, patterns and frameworks. Businesses also seek techniques to manage the complexity of systems as they increase in scope and scale. In particular, they recognize the need to solve recurring architectural problems, such as physical distribution, concurrency, replication, security, load balancing and fault tolerance. Additionally, the development for the World Wide Web, while making some things simpler, has exacerbated these architectural problems. The Unified Modeling Language (UML) was designed to respond to these needs and to supply the programmers and the project managers with a visual application state and layout.
There isn't a clear why to implement the usage of UML for rails development, but here are the tools i did find.
- RailsRoad is a RailRoad is a class diagrams generator for Ruby on Rails applications. It's a Ruby script that loads the application classes and analyzes its properties (attributes, methods) and relationships (inheritance, model associations like has_many, etc.) The output is a graph description in the DOT language, suitable to be handled with tools like Graphviz. Last tested on old rails versions!
- A visual paradigm plugin for generating ruby (yap, not rails)
- ruby-uml, couldn't find any documentations.
- The generate_from_uml plugin does the oppsite and enerates rails models from a UML schema, i didn't see how advanced relations are handled though (:has_many :through for example)
Deploying Ruby on Rails on EC2 - Deploying Oracle
Well, generally i use Oracle for my large scale applications.
Some people might say that it's not too-competible with Rails, but using the oracle_enhanced_adapter didn't pop up any special issues (it even supports Oracle's bind variables technique).
The Oracle Cloud Deployment tutorials on Oracle's website contains explanations, PDFs, tutorials and demos on how and when to deploy Oracle DB in the cloud.
Extra guides include backup and recovery practices.
Web Base SQL schema design
This amazing online SQL design (ERD) tool called wwwsqldesigner, is a great solution for those of us who get too troubled by designing simple apps database layouts. (NOTE: you should always hire a professional DBA)
Checkout the Homepage on GoogleCode, and Live demo.
Deploying Ruby on Rails on EC2 - Update
Tom Mornini, the Founder and CTO of EngineYard, introduced me their latest announcement, Solo.
Solo is an inexpensive, web-based platform for on-demand management of your Ruby on Rails web application on Amazon’s cloud computing infrastructure. Manage your web application from development to deployment. You get Engine Yard’s Ruby on Rails deployment expertise wrapped in an easy-to-use interface.
Solo removes a lot of the hassles and provides a battle tested Rails stack and many tools required to create reliable and manageable deployment atop Amazon's wonderful AWS offerings.
Tom also mentions that using my Deploying on Ruby on Rails on EC2 guide is not recommended for production stages and that users on AWS should always keep in mind that in case of a crash or reboot of the environment will result in lost data (without proper countermeasures, like EngineYard offers)
So your setup scenario as i suggested, should be used for development only, and carefully even in that case and keep in mind the non-persistence state of EC2 instance disks.
Beautiful Javascript Date Pickers
Source: Woork! - Beautiful Javascript Date Pickers
Great list of Date Picker plugins and scripts, for several Javascript frameworks.
Woork: 10 Javascript UI Libraries
Source: Woork!

IT Mill Toolkit is an open-source framework, providing widgets and tools for the development of Rich Internet Applications (RIAs). Deliver web applications without worrying about incompatibilities of web browsers, DOM or JavaScript by using standard Java tools.

LivePipe UI is a suite of high quality widgets and controls for web 2.0 applications built using the Prototype JavaScript Framework. Each control is well tested, highly extensible, fully documented and degrades gracefully for non JavaScript enabled browsers where possible

Iwebkit is the revolutionnairy kit used to create high quality iPhone and iPod touch websites in a few minutes. In the first 4 months of it's existance the pack has greatly evolved from a basic idea to a project that has reached worldwide fame!

Jitsu contains an integrated set of tools to enable developers to build and deploy sophisticated user interfaces for web applications. These include an Xml markup language, page compiler, data binding engine, JavaScript runtime, control library, runtime inspector, animation engine, cross-platform library, Ajax, and back button support.

MochaUI is a web applications user interface library built on the Mootools JavaScript framework.Uses: web applications, web desktops, web sites, widgets, standalone windows and modal dialogs.
6. Echo Web Framework
Echo is an open-source framework for developing rich web applications. From the developer's perspective, Echo behaves as a user interface toolkit--like Swing or Eclipse SWT. AJAX technology is employed to deliver a user experience to web clients that approaches that of desktop-based applications.

The YUI Library is a set of utilities and controls, written in JavaScript, for building richly interactive web applications using techniques such as DOM scripting, DHTML and AJAX. YUI is available under a BSD license and is free for all uses.
8. Sigma Ajax UI builder
Written in javascript and PHP, SigmaVisual is web based visual AJAX UI builder for professional developers. Developers save their time in building up prototype as well as real web applications. TreeBar, TreeGrid, Layout, Menu are supported.

WUI (Web User Interface) is an MVC framework for writing web UIs in a single language: Java. Write web apps with components, widgets & events, not JSPs. Runs in any servlet 2.3 container. Similar to Model 2 / Struts, only better. Apache-style license.
10. Butterfly Web UI
Butterfly Web UI is a component oriented web framework for Java, like Wicket or Tapestry. The main advantage compared to these frameworks is that Butterfly Web UI integrates naturally with Butterfly DI Container, giving you a state-of-the-art dependency injection container to help you structure and decouple the internal components of your web applications.
Yet another Framwork: Pylons
Pylons combines the very best ideas from the worlds of Ruby, Python and Perl, providing a structured but extremely flexible Python web framework. It's also one of the first projects to leverage the emerging WSGI standard, which allows extensive re-use and flexibility — but only if you need it. Out of the box, Pylons aims to make web development fast, flexible and easy
Looks like a fun language, after reading the Pylon book for a few days now, it looks very interesting.
This framework is based on Paste and allows some extended python usage, templating, modeling and basically a full MVC application development interface (even CouchDB).
What i can say about it, is that it's some kind of another Rails-ish framwork ("paster" for "generate" for example), these frameworks are popping like mushrooms these days (Currently reviewing the PHP Akelos framework as well)
The pylons web site offers general information, a link to the online book and some screencasts.
Browser Security Hand book
The Browser Security Handbook is a free and no-nonsense guide to the security concepts in today's main browsers. It covers all security features, explains why and in which browsers certain attack methods work and talks about experimental security mechanisms in browsers. It's a recommended read for everyone who creates web applications.
Important read, i remember reading a similar article a few years back (FF1 vs. IE6) and i learned some good stuff.
Get it here
Rails 2 i18n: Globalize2 plugin for Rails
A while ago i posted a post about a Globalize plugin bug in Rails 2.x. Eventually i found a solution but i was still waiting for a real solution for Rails 2.x to supersede my monkey patching.
Well, it's here.
note for the Hebrew natives: check out the examples :)
Globalize2 is the successor of Globalize for Rails.
It is compatible with and builds on the new I18n api in Ruby on Rails. and adds model translations as well as a bunch of other useful features, such as Locale fallbacks (RFC4647 compliant) and automatic loading of Locale data from defined directory/file locations.
Globalize2 is much more lightweight and modular than its predecessor was. Content translations in Globalize2 use default ActiveRecord features and do not limit any functionality any more.
All features and tools in Globalize2 are implemented in the most unobstrusive and loosely-coupled way possible, so you can pick whatever features or tools you need for your application and combine them with other tools from other libraries or plugins.
Get it from joshmh Github Repo
Elliott Kember: Sexy Page Curl JQuery plugin
Just one of those fun-and-no-real-use things you might want to put in your blog/personal-homepage.
Elliott Kember created this Page Curl plugin for JQuery which shows a page curl on the corner of your website, with the option to set an image below it.
Check the demos.
Rails Migrations - Running a Single Migration
We all know the good old
rake db:migrateAnd we all know the Rails 2.x
rake db:migrate:rollbackto go back in time and fix what we did.
Now, in Rails 2.x you can specify a single migration by specifing one of the each
rake db:migrate:up VERSION=<migration_timestamp> # Runs the self.up rake db:migrate:down VERSION=<migration_timestamp> # Runs the self.downNote that migrations are meant to be concurrent and that's the most basic purpose in which they are there for, executing an out of line migration may cause you problems in the future, so try to stay as clean as possible when dealing with migrations
CSS Sprites: onilne sprite generator
The CSS sprites technique is basically a technique that reduces the CSS requests when dealing with background images.
Example: Lets say you have 2 selectors in your CSS,
div#first { background: url(first_bg.png); } div#second { background: url(second_bg.png); }
when loading your CSS file will preform 2 requests, one for each image you specified as background, so when dealing with a complete design, you are probably talking about 40-50+ image requests as such.
CSS sprites solves this problem by loading only one image, that contains all the images you will use on your site within, and displaying the correct image by playing with a combination of a background offset (background-position CSS attribute) and the size of the element.
This technique can be very effective for improving site performance, particularly in situations where many small images, such as menu icons, are used. The Yahoo! home page, for example, employs the technique for exactly this.
Examples and full explanation can be found here at the CSS Tricks.com - CSS Sprites: HowTo or here at the List Apart: Image Slicing's kiss of death article.
Today, i found a Sprite image generator that actually generates this Sprite image file from a zip file you upload and containing all the images, and produces the image as said before, but also the output CSS statements.
very useful.
Firefox vs. Explorer: the power of free will
First i have to clear the background. In Israel, like every other place in the world, IE still rules. The problem in Israel is that there is not enough exposure for other browsers, and even to other OS besides windows. People are not familiar with Ubuntu (almost) at all, and the Macs are pretty expensive in Israel, relatively a lot more than it is elsewhere.
This causes some serious problems in the Israeli online experience, government and other public service provider websites can not be viewed in other browsers except IE, and the web developer community is in a consistant battle to explain to some low-level yet common development firms, why you should implement web standards and support other browsers.
Courses and training programs are more than often don't approach standards and semantic HTML issues, but there are numerous which hold ActiveX in their syllabuses
Back to business, that guy explained that
"People who don't use IE are a minority and if they choose to handicap themselves, it's not my or the government responsibility to enable the support for other browsers".
What can you say about that?
Putting a side everything i have against Microsoft as a corporation, i think that IE is the handicaped browser, not Firefox/Opera/every other browser.
Handicap is not a plugin and extenstion enabled browser, it's the browser that can't support 40% of the W3C Standard for example.
You can find too many hits on why you should dump IE(#2, #3 so on..) and i don't think it's the Firefox hype talking, it's simply the people who had enough with their browser doing stuff he shouldn't do, and not what they want or asked it to do.
BTW, i ended that discussion with that guy... you can't argue with someone that can't understand the meaning of the things you say, just like one of the other forum users said: "Arguing the benefits of Firefox over IE with an iE user is like arguing the advantages of a flashlight with a caveman carrying a torch."
ActiveRecord.js: a Relational js ActiveRecord implementation
ActiveRecord.js is the first released component of ActiveJS a JavaScript framework initiative sponsored by Aptana. This project is still in it's early stages and we welcome your thoughts and ideas on the future of JavaScript application development. Since this is a GitHub hosted project, if you would like to directly contribute we recommend you fork the project and initiate a pull request to merge your changes
The ActiveRecordjs Homepage
Not tested in IE initiative: a new savethedevelopers.com
savethedevelopers.com used to be a great script that pops up when the client's browser was IE6, warning him about the mischief ways of that browser and urging the user to either update to IE7 or go the happy road on Firefox.
Than, something weird happend.
The website stopped working and now redirects to Microsoft's IE download page... yak.
The "not tested in IE initiative" tries to re-incarnate that thought. So if you are unsure that your website will be displayed correctly on IE6, use one of these icons link to the project's home page.
- 142x50 pixels :
- 85x30 pixels :
- 57x50 pixels :
- 34x30 pixels :
Tools of the Trade: Web development tools list
Well, it's not mine, but it's great.
Dion Almaer's Web resources page includes a shiny list of web resources/tools/tips he uses when developing web applications (on PHP though, baaah.).
He indicates that some of the links are outdated, but promises to fix it. as soon as possible.
All Browsers Online Preview
Great tool, shows you how your website looks in many browsers and OS's, including screen shots.
Visit at browsershots.org
Rails and Amazon EC2 - Beginners guide
First of all, Amazon AWS rocks. it's a great, stable and not so expensive way to get your application up and running, but also ready for any disaster to come (Someone said Digg effect?).
Getting your application on to Amazon EC2 is not as complicated as you think, managing and controlling your instances may require a professional system administrator, but i would recommend that anyway (except if you go and host your application on EngineYard than you are worry-free, but you'll pay.. ohh you'll pay for that sense of security).
Get your application Ready
There is no real need to setup your EC2 instances in day 1, you can wait until the application is mature enough to be deployed onto a production infrastructure. Amazon EC2 costs per usage, so, it will cost you to have your application up and running on EC2, keep that in mind (although the prices are a joke).
Setting things up
Once your application is ready to be deployed to EC2, you'll need an image.
Image, in EC2 terms, is a reference to an OS + all required installations and configuration needed to run your application.
The best all-round image to use with rails is Pawl Dowman's Rails on EC2 bundle (or here). EC2onRails is great. Unfortunately, if you’ve never used EC2 before, you probably won’t be able to “Deploy a Ruby on Rails app on EC2 in Five Minutes” as the documentation claims, so this document will try to fill in the gaps for someone who has never worked with EC2.
note: it's never too early to sit down and read Amazon's documentations, they are not that bad
Local machine
First thing is first, your development machine, the one from which you will be deploying to EC2 should undoubtably hold:
- Ruby on Rails (do yourself a favor, at least version 2.1)
- MySQL (Well, we are going to work with RailsonEC2, which includes it, if you need another db.. you'll need another image).
- Java Development Kit 1.5 or later installed.
Mac users should be ready-to-go (Hah! Hah!), Windows/Linux users if you don’t have it, download it from Sun's website.
Make sure to download a version labeled “JDK”.
Java is required for the tools that Amazon provides to manage EC2 instances. (rumor says that there are ruby management tools also, but i prefer the ones that Amazon gives).
Once you are set with these issues, you'll need to sign up for the Amazon EC2 service, do that right here.
Now, sign up for the S3 service.
Why? becaous althougn your image (later: 'instance') includes storage, images/instances can be brought down, up, dropped and even deleted, that will ultimatly, kill your data.
S3 is Amazon’s “Simple Storage Service”. S3 is a super-inexpensive service to store files into “buckets in the cloud”. S3 will be used for database backups of your Rails Application.
Sign up for S3 here.
What you'll need to keep somewhere
After signing up, you will need to collect four pieces of information from your AWS account by visiting the Access Identifiers page.
- Your account number. The account number can be found at the upper right of the Access Idenfiers page and should be three four-digit numbers separated by dashes.
- Your Access Key ID. This is a 20 or so character key found in a beige box a little below your account number.
- Your Secret Access Key. This is a 40 or so character key found in another beige box just below your Access Key ID. If this is your first time on this page, you may have to generate your key. Click the “Show” button to display your 40 character key.
- Your X.509 Certificate Create and download the X.509 certificate below the Secret Access Key section. Place the public and private keys into a folder called “.ec2″ in your home directory.
Download and Install the EC2 Command Line Tools
The EC2 command-line tools are a Java-based set of tools that allow you to create and manage machine instances on EC2.
Download the Command Line ToolsDownload here Amazon EC2 Command-Line Tools and extract the zip file, remember where you put it, i always keep it in my home folder as a hidden folder named .ec2 (/Users/eizesus/.ec2/ on OSX).
Set Appropriate Environment Variables
export EC2_PRIVATE_KEY=/Users/eizesus/.ec2/pk-5xxxxxxxx7.pem export EC2_CERT=/Users/eizesus/.ec2/cert-5xxxxxxxxxxxxx7.pem export EC2_HOME=/Applications/java/ec2-api-tools-1.3-24159 export PATH=$EC2_HOME/bin:$PATHRemember to replace my library with yours.
I suggest adding these lines to your startup profile, whatever it maybe on your OS. (OSX, it's in /etc/profile).
After you do that, reload your session (close the console/command line and open it again).
Create a Key Pair the Deployment machine
ec2-add-keypair my-secret-codeThe string my-secret-code can and should be anything that you like, try to pick something that makes sense for your setup (remember that i will keep on writing my-secret-code, use your phrase instead).
Save the output from that into a file named id_rsa-my-secert-code and paste everything between (and including) the “—–BEGIN RSA PRIVATE KEY—–” and “—–END RSA PRIVATE KEY—–” lines into it. Confirm that the file contents looks exactly like this, then save the file into the file:
~/.ssh/id_rsa-my-secret-code
Set permissions on the new key file:
chmod 600 ~/.ssh/id_rsa-my-secret-code
Start Up an Amazon EC2 Instance
An Amazon Machine Image (AMI) is a named configuration of an EC2 image.The current AMI id’s for EC2onRails are:
- ami-c9bc58a0 (32-bit)
- ami-cbbc58a2 (64-bit)
ec2-run-instances ami-c9bc58a0 -k my-secret-codeThe second line of the results returned will look like:
INSTANCE i-XXXXXXXX ami-c9bc58a0 pending my-secret-code 0The pending my-secret-code means that the image is pending.
To check the status of the instance build type:
ec2-describe-instances i-XXXXXXXXReplace the i-XXXXXXXX above with the string that comes after INSTANCE in the second line of the results from the ec2-run-instances command.
Run it again until it says running my-secret-code, than you have an Amazon EC2 instance running! (yippie!).
Take note of the string that looks like
ec2-xx-xxx-xxx-xx.compute-1.amazonaws.comthat is your machine’s address.
Authorize SSH and HTTP
ec2-authorize default -p 22should result in:
PERMISSION default ALLOWS tcp 22 22 FROM CIDR 0.0.0.0/0
ec2-authorize default -p 80This should return something like:
PERMISSION default ALLOWS tcp 80 80 FROM CIDR 0.0.0.0/0
you are up and ready to go!
You should be able to ssh into the your new machine, replace
ec2-xx-xxx-xxx-xx.compute-1.amazonaws.com
with your own machine’s address:
ssh -i ~/.ssh/id_rsa-ec2-rails-keypair root@ec2-xx-xxx-xxx-xx.compute-1.amazonaws.comYou may have to type “yes” to accept the authenticity of the host.
Prepare Your Application for EC2
EC2 for Rails requires Capistrano:sudo gem install capistrano -v 2.4.3And then install the EC2 for Rails gem:
sudo gem install ec2onrailsOkay, you need to add three configuration files to your Rails application.
- Capfile - save this file at the root of your application.
- deploy.rb - save this file in the /config folder.
- s3.yml - also save this file in the /config folder.
Customize the EC2 Configurations
capfile can an be left as-downloaded.
config/s3.yml
- This configuration is pretty simple, under the production section, put your AWS information that you noted above. aws_access_key: (you got me when you signed up) aws_secret_access_key: (me too) bucket_base_name: production.yourname.comconfig/deploy.rb - There are a lot of definitions to be made, read all the comments and setup the deploy process as your applicaiton requires.
config/database.yml - For the production section, basically add any good password that you like and add hostname: db_primary. It should look something like:
production: adapter: mysql encoding: utf8 database: appname_production username: user (not ROOT!) password: password (no, no empty passwords) hostname: db_primary
Run the EC2 on Rails Capistrano Tasks
cap ec2onrails:get_public_key_from_server cap ec2onrails:server:set_roles
Configure the db and stuff:
cap ec2onrails:setupAnd launch your app:
cap deploy:coldAnd wooooohoooo, check your application url.
That's it basically, you are up.
Twiters Around
About Me
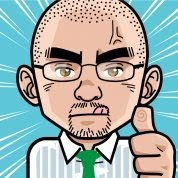
- Elad Meidar
- I am a web developer for more than 9 years, managed, cried, coded, designed and made money in this industry. now trying to do it again.
Blog Archive
-
▼
2009 (67)
-
▼
March (9)
- Moved to Mephisto
- Rails Nested Resources Tutorial
- Raphael: Amazing vector graphics Javascript Libraty
- Storing Files in Ruby on Rails
- Live Free: Open Source Software indexes
- Debugging Javascript in Web Applications: Major br...
- 140 Characters Web applications
- Rails and Facebook: Why (still) iFrame is better t...
- Rails Facebook App Enlightments: HTTP timeouts
-
►
February (14)
- Rant: Why i Hate Facebook (API)
- Startup Thoughts: Realty and a Dream
- Startup Thoughts: Sharing the cake
- The Lazy Web Developer: web 2.0 generators list
- How to avoid screwing up a software project
- Rails 2.3: Weird multipart form bug
- Update: Social Network Ruby on Rails Applications
- Mockups: Easily create application mockups
- FireFox blogging extention: ScribeFire
- Rails Conditional Eager Loading
- 2 Useful CSS tips
- IE8 RC1: IE6 again?
- CSS Frameworks Tutorials and getting started guides
- Ruby 1.9 is out
-
►
January (44)
- Amazon AWS: Calculate your monthly costs on Amazon...
- Noupe: 15+ Incredibly Useful Mac Apps For Freelan...
- UML for Rails applications
- Deploying Ruby on Rails on EC2 - Deploying Oracle
- Web Base SQL schema design
- Deploying Ruby on Rails on EC2 - Update
- Beautiful Javascript Date Pickers
- Woork: 10 Javascript UI Libraries
- Yet another Framwork: Pylons
- Browser Security Hand book
- Rails 2 i18n: Globalize2 plugin for Rails
- Elliott Kember: Sexy Page Curl JQuery plugin
- Rails Migrations - Running a Single Migration
- CSS Sprites: onilne sprite generator
- Firefox vs. Explorer: the power of free will
- ActiveRecord.js: a Relational js ActiveRecord impl...
- Not tested in IE initiative: a new savethedevelope...
- Rails Testing: Getting started free e-book
- Tools of the Trade: Web development tools list
- All Browsers Online Preview
- Rails and Amazon EC2 - Beginners guide
-
▼
March (9)
Labels
- 1.9 (1)
- 2.0 (1)
- 2.2 (1)
- 2.3 (1)
- 2.x (1)
- accessibility (2)
- account (1)
- actionmailer (1)
- activerecord (3)
- adsense (1)
- affiliate (1)
- ajax (3)
- amazon (4)
- analytics (1)
- api (3)
- application (1)
- array (1)
- associations (1)
- attachment_fu (1)
- autocomplete (1)
- aws (2)
- blog (4)
- books (4)
- boolean (1)
- browser (8)
- browsers (3)
- bugs (4)
- buzz (1)
- callback (1)
- callbacks (1)
- caller (1)
- capistrano (1)
- chronic (1)
- class (2)
- classes (1)
- classifieds (1)
- client (1)
- coding (2)
- collboration (1)
- console (2)
- convert (1)
- core (2)
- core. object (1)
- cost (1)
- css (7)
- database (10)
- date (3)
- dating (1)
- db (2)
- debug (2)
- deploy (5)
- deployment (3)
- design (11)
- development (5)
- dojo (1)
- eager (1)
- ec2 (2)
- effects (1)
- elad (2)
- email (3)
- engine (1)
- engineyard (1)
- english (1)
- entrepreneur (1)
- environment (2)
- erd (1)
- error (1)
- example (1)
- expire (1)
- explorer (1)
- extention (1)
- extra (2)
- facebook (2)
- fast (1)
- file (1)
- files (1)
- firefox (4)
- fixtures (1)
- font (1)
- form (1)
- framework (16)
- free (6)
- funny (2)
- gem (7)
- gems (2)
- git (5)
- globalize (2)
- gmail (3)
- google (8)
- graphes (2)
- graphics (1)
- guides (4)
- hacks (1)
- hosting (1)
- html (1)
- http (1)
- ie (5)
- ie8 (1)
- image (2)
- indomite (1)
- install (1)
- iphone (1)
- israel (2)
- javascript (19)
- JQuery (10)
- js (3)
- legacy (1)
- leopard (1)
- lib (1)
- library (3)
- links (2)
- list (1)
- loading (1)
- log (1)
- logger (2)
- logging (1)
- love (1)
- mac (4)
- mail (2)
- manuals (1)
- mapping (1)
- mass (1)
- mephisto (3)
- mercurial (1)
- meta (1)
- metaprogramming (3)
- microsoft (1)
- migrations (2)
- minimagick (1)
- mistake (1)
- mistakes (1)
- model (1)
- money (4)
- mootools (4)
- move (1)
- movies (2)
- music (1)
- mvc (2)
- mysql (2)
- native (1)
- nested (1)
- network (1)
- new (1)
- newsletter (1)
- num_to_english (1)
- numbers (2)
- object (1)
- observer (1)
- ohad (1)
- open (1)
- operators (1)
- optimization (4)
- oracle (4)
- overload (1)
- parse (1)
- pdf (4)
- php (3)
- plugin (11)
- plugins (7)
- programming (4)
- programs (1)
- projects (5)
- prototype (1)
- queries (1)
- rails (50)
- rails 2.0 (10)
- rails 2.1 (6)
- rake (2)
- rants (1)
- record (1)
- reflections (1)
- release (3)
- rendering (1)
- reset (1)
- resize (1)
- rest (1)
- rjs (1)
- ror (1)
- routes (3)
- routes.rb (2)
- ruby (49)
- ruby on bells (2)
- ruby on rails (28)
- ruby-debug (1)
- s3 (2)
- salary (1)
- scaling (2)
- schema (2)
- screencasts (1)
- script (4)
- scripts (1)
- search engine optimization (1)
- security (3)
- seeking alpha (1)
- select (1)
- sendmail (1)
- seo (2)
- sequencer (1)
- sessions (1)
- share (2)
- shell (1)
- site (3)
- site map (1)
- sites (1)
- skype (1)
- social (2)
- source (1)
- source control (4)
- sprites (1)
- sql (1)
- stand alone (1)
- standards (1)
- startup (6)
- storage (2)
- subversion (4)
- svn (1)
- team (1)
- technology (1)
- testing (1)
- textmate (1)
- thread (1)
- time (1)
- tips (45)
- tools (5)
- tracking (1)
- tricks (36)
- tutorials (5)
- ui (4)
- uml (1)
- update (1)
- upgrading (2)
- url (1)
- usability (2)
- user interface (7)
- validations (1)
- vector (1)
- vote (1)
- web (9)
- web services (2)
- web2.0 (4)
- webistrano (1)
- wire (1)
- wwr (1)
- xhtml (1)
- yahoo (1)
- yui (1)
- רובי (8)
- רובי און ריילס (7)