Rails's eager loading (ScreenCast) is an important practice that saves some requests and queries when dealing with table relations in rails.
Up until now, using a condition hash to specify query was a little tricky if you use the eager loading method:
User.find(:all, :include => :jobs, :conditions => ["jobs.salary > ?",1000])
or using a hash (without eager loading)
User.find(:all, :conditions => {:name => 'eizesus'})
Using a conditions array/hash isn’t always my favorite way to write finds in Rails, but in certain cases must have when you deal with complex queries (like and "Advanced Search" feature).
In my current work,I needed to run conditions on an associated table in just such an "Advanced search" feature, so i came up with this simple and easy way to use a condition hash for eager loading methods:
User.find(:all, :include => :jobs, :conditions => {:name => 'eizesus', :jobs => {:position => 'Manager'})
or you can prepare the condition hash on the fly:
conditions = {}
conditions[:first_name] = params[:first_name] unless params[:first_name].blank?
conditions[:last_name] = params[:last_name] unless params[:last_name].blank?
conditions[:jobs] = {:position => params[:position]} unless params[:position].blank?
@users = User.find(:all, :conditions => conditions, :include => :jobs)
Ruby On Rails and a Conning Israeli entrepreneur
Rails Conditional Eager Loading
Ruby 1.9 is out
source Ruby Inside
Benchmarks say it's twice as fast as 1.8.7, but developers worn not to use it as a production ruby rght away becouse it may a lot of libraries and Gems.
so, be worned.
UML for Rails applications
UML is a Graphical language for visualizing, specifying and constructing the artifacts of a software-intensive system. The Unified Modeling Language offers a standard way to write a system's blueprints, including conceptual components such as business processes and system functions as well as concrete things such as programming language statements, database schemas, and reusable software components.[1] UML combines the best practice from data modeling concepts such as entity relationship diagrams, business modeling (work flow), object modeling and component modeling. It can be used with all processes, throughout the software development life cycle, and across different implementation technologies.
As the strategic value of software increases for many companies, the industry looks for techniques to automate the production of software and to improve quality and reduce cost and time-to-market.
These techniques include component technology, visual programming, patterns and frameworks. Businesses also seek techniques to manage the complexity of systems as they increase in scope and scale. In particular, they recognize the need to solve recurring architectural problems, such as physical distribution, concurrency, replication, security, load balancing and fault tolerance. Additionally, the development for the World Wide Web, while making some things simpler, has exacerbated these architectural problems. The Unified Modeling Language (UML) was designed to respond to these needs and to supply the programmers and the project managers with a visual application state and layout.
There isn't a clear why to implement the usage of UML for rails development, but here are the tools i did find.
- RailsRoad is a RailRoad is a class diagrams generator for Ruby on Rails applications. It's a Ruby script that loads the application classes and analyzes its properties (attributes, methods) and relationships (inheritance, model associations like has_many, etc.) The output is a graph description in the DOT language, suitable to be handled with tools like Graphviz. Last tested on old rails versions!
- A visual paradigm plugin for generating ruby (yap, not rails)
- ruby-uml, couldn't find any documentations.
- The generate_from_uml plugin does the oppsite and enerates rails models from a UML schema, i didn't see how advanced relations are handled though (:has_many :through for example)
Rails and Amazon EC2 - Beginners guide
First of all, Amazon AWS rocks. it's a great, stable and not so expensive way to get your application up and running, but also ready for any disaster to come (Someone said Digg effect?).
Getting your application on to Amazon EC2 is not as complicated as you think, managing and controlling your instances may require a professional system administrator, but i would recommend that anyway (except if you go and host your application on EngineYard than you are worry-free, but you'll pay.. ohh you'll pay for that sense of security).
Get your application Ready
There is no real need to setup your EC2 instances in day 1, you can wait until the application is mature enough to be deployed onto a production infrastructure. Amazon EC2 costs per usage, so, it will cost you to have your application up and running on EC2, keep that in mind (although the prices are a joke).
Setting things up
Once your application is ready to be deployed to EC2, you'll need an image.
Image, in EC2 terms, is a reference to an OS + all required installations and configuration needed to run your application.
The best all-round image to use with rails is Pawl Dowman's Rails on EC2 bundle (or here). EC2onRails is great. Unfortunately, if you’ve never used EC2 before, you probably won’t be able to “Deploy a Ruby on Rails app on EC2 in Five Minutes” as the documentation claims, so this document will try to fill in the gaps for someone who has never worked with EC2.
note: it's never too early to sit down and read Amazon's documentations, they are not that bad
Local machine
First thing is first, your development machine, the one from which you will be deploying to EC2 should undoubtably hold:
- Ruby on Rails (do yourself a favor, at least version 2.1)
- MySQL (Well, we are going to work with RailsonEC2, which includes it, if you need another db.. you'll need another image).
- Java Development Kit 1.5 or later installed.
Mac users should be ready-to-go (Hah! Hah!), Windows/Linux users if you don’t have it, download it from Sun's website.
Make sure to download a version labeled “JDK”.
Java is required for the tools that Amazon provides to manage EC2 instances. (rumor says that there are ruby management tools also, but i prefer the ones that Amazon gives).
Once you are set with these issues, you'll need to sign up for the Amazon EC2 service, do that right here.
Now, sign up for the S3 service.
Why? becaous althougn your image (later: 'instance') includes storage, images/instances can be brought down, up, dropped and even deleted, that will ultimatly, kill your data.
S3 is Amazon’s “Simple Storage Service”. S3 is a super-inexpensive service to store files into “buckets in the cloud”. S3 will be used for database backups of your Rails Application.
Sign up for S3 here.
What you'll need to keep somewhere
After signing up, you will need to collect four pieces of information from your AWS account by visiting the Access Identifiers page.
- Your account number. The account number can be found at the upper right of the Access Idenfiers page and should be three four-digit numbers separated by dashes.
- Your Access Key ID. This is a 20 or so character key found in a beige box a little below your account number.
- Your Secret Access Key. This is a 40 or so character key found in another beige box just below your Access Key ID. If this is your first time on this page, you may have to generate your key. Click the “Show” button to display your 40 character key.
- Your X.509 Certificate Create and download the X.509 certificate below the Secret Access Key section. Place the public and private keys into a folder called “.ec2″ in your home directory.
Download and Install the EC2 Command Line Tools
The EC2 command-line tools are a Java-based set of tools that allow you to create and manage machine instances on EC2.
Download the Command Line ToolsDownload here Amazon EC2 Command-Line Tools and extract the zip file, remember where you put it, i always keep it in my home folder as a hidden folder named .ec2 (/Users/eizesus/.ec2/ on OSX).
Set Appropriate Environment Variables
export EC2_PRIVATE_KEY=/Users/eizesus/.ec2/pk-5xxxxxxxx7.pem export EC2_CERT=/Users/eizesus/.ec2/cert-5xxxxxxxxxxxxx7.pem export EC2_HOME=/Applications/java/ec2-api-tools-1.3-24159 export PATH=$EC2_HOME/bin:$PATHRemember to replace my library with yours.
I suggest adding these lines to your startup profile, whatever it maybe on your OS. (OSX, it's in /etc/profile).
After you do that, reload your session (close the console/command line and open it again).
Create a Key Pair the Deployment machine
ec2-add-keypair my-secret-codeThe string my-secret-code can and should be anything that you like, try to pick something that makes sense for your setup (remember that i will keep on writing my-secret-code, use your phrase instead).
Save the output from that into a file named id_rsa-my-secert-code and paste everything between (and including) the “—–BEGIN RSA PRIVATE KEY—–” and “—–END RSA PRIVATE KEY—–” lines into it. Confirm that the file contents looks exactly like this, then save the file into the file:
~/.ssh/id_rsa-my-secret-code
Set permissions on the new key file:
chmod 600 ~/.ssh/id_rsa-my-secret-code
Start Up an Amazon EC2 Instance
An Amazon Machine Image (AMI) is a named configuration of an EC2 image.The current AMI id’s for EC2onRails are:
- ami-c9bc58a0 (32-bit)
- ami-cbbc58a2 (64-bit)
ec2-run-instances ami-c9bc58a0 -k my-secret-codeThe second line of the results returned will look like:
INSTANCE i-XXXXXXXX ami-c9bc58a0 pending my-secret-code 0The pending my-secret-code means that the image is pending.
To check the status of the instance build type:
ec2-describe-instances i-XXXXXXXXReplace the i-XXXXXXXX above with the string that comes after INSTANCE in the second line of the results from the ec2-run-instances command.
Run it again until it says running my-secret-code, than you have an Amazon EC2 instance running! (yippie!).
Take note of the string that looks like
ec2-xx-xxx-xxx-xx.compute-1.amazonaws.comthat is your machine’s address.
Authorize SSH and HTTP
ec2-authorize default -p 22should result in:
PERMISSION default ALLOWS tcp 22 22 FROM CIDR 0.0.0.0/0
ec2-authorize default -p 80This should return something like:
PERMISSION default ALLOWS tcp 80 80 FROM CIDR 0.0.0.0/0
you are up and ready to go!
You should be able to ssh into the your new machine, replace
ec2-xx-xxx-xxx-xx.compute-1.amazonaws.com
with your own machine’s address:
ssh -i ~/.ssh/id_rsa-ec2-rails-keypair root@ec2-xx-xxx-xxx-xx.compute-1.amazonaws.comYou may have to type “yes” to accept the authenticity of the host.
Prepare Your Application for EC2
EC2 for Rails requires Capistrano:sudo gem install capistrano -v 2.4.3And then install the EC2 for Rails gem:
sudo gem install ec2onrailsOkay, you need to add three configuration files to your Rails application.
- Capfile - save this file at the root of your application.
- deploy.rb - save this file in the /config folder.
- s3.yml - also save this file in the /config folder.
Customize the EC2 Configurations
capfile can an be left as-downloaded.
config/s3.yml
- This configuration is pretty simple, under the production section, put your AWS information that you noted above. aws_access_key: (you got me when you signed up) aws_secret_access_key: (me too) bucket_base_name: production.yourname.comconfig/deploy.rb - There are a lot of definitions to be made, read all the comments and setup the deploy process as your applicaiton requires.
config/database.yml - For the production section, basically add any good password that you like and add hostname: db_primary. It should look something like:
production: adapter: mysql encoding: utf8 database: appname_production username: user (not ROOT!) password: password (no, no empty passwords) hostname: db_primary
Run the EC2 on Rails Capistrano Tasks
cap ec2onrails:get_public_key_from_server cap ec2onrails:server:set_roles
Configure the db and stuff:
cap ec2onrails:setupAnd launch your app:
cap deploy:coldAnd wooooohoooo, check your application url.
That's it basically, you are up.
Looking for a RoR team
Well, it's time to move on and work on marketing my new application (can't tell you what it is yet :) ), but i need a dedicated, professional Rails+Client Side development team to support the final stage of development, and to continue supporting further changes.
I heard about people getting teams in India, and some companies offer services for out-sourcing code, but i prefer at the moment to find a team (india, USA doesn't matter) that will support my application and will be ready to put some real work into it.
anyway, if anyone is interested, post me a message on linkedin.
basically the skills i need are
* Ruby on Rails experience (2.x)
* Client side (JQuery, MooTools, MochaUi is a great plus).
* CSS and some basic design capabilities.
* DBA (MySQL, Oracle experience will be a great advantage).
* Amazon AWS+S3+EC2 experience.
* ability to work remotely.
i will prefer a whole team, rather than finding these skills in specific programmers and building the team myself.
UPDATE: All positions are filled, thank you everyone!
Observers, Associations and Callbacks
In my latest project, i need to notify a user via email about a certain kind of instance being created, so far normal.
This instance is created with a several of associated instances, let's say i am creating an article instance with linked categories using the virtual attribute technique (RailsCasts #16).
I created an observer for the article class to send an email with the article and categories after a successful creation of an article instance, therefore i immediately assumed that the correct callback is after_create. well, it's not.
when i used after_create, the email arrived with an empty list of categories, almost like they weren't saved, but a short trip to the console showed that the categories were created and that they are associated to the article as i wanted.
The problem resides in the order rails does this nested object creation, first the initial object is being created (the artical) and only than the association are created (after i used #build, watch the screen cast!), which causes the email to be triggered one step earlier than i wanted.
after finding this article i changed the observer's callback to after_save which apperantly is being triggers after the associations are saved as well.
i spent a lot of time on it, hope this helps.
Moving to Mephisto (hopefully)
i have had it with this blogger account, you can say a lot of good things about this blog platform, but it is still a shared blog platform and it's not very professional to store you crown jewel on a blog platform which is written in Python :).
So i decided, i am moving to Mephisto. Mephisto is a Ruby on Rails blog platform and a self proclaimed "Best blog platform ever". well, i don't really know if that is true or not, but after checking it out locally i decided to use Mephisto for my permanent new blog.
The only problem i have right now, is how to transfer my Blogger posts/comments into my brand new Mephisto database. Mephisto is equipped with a "converstion" tools from Wordpress and Movable-type and as far as i know, nothing for blogger.
the blogger draft service (experimental blogger interface) enables you to download your entire post and comment history as XML so i might try to simply parse it into the DB (Or write a Converter).
Anyway, i'll be kinda busy doing it in the near future, so probably no posts in the near future.
Rails 2.2: Getting Started link list
General Documentation and Guides
From railsinside.com
Rails 2.2 Release Notes - A very solid set of release notes for 2.2 with basic coverage of the new features (with short code examples and links) as well as a list of deprecated features. They were compiled by Ruby / Rails Inside's very own Mike Gunderloy!
Upgrading RubyGems to 1.3.x - Depending on your setup, Rails 2.2 may demand that you upgrade to RubyGems 1.3.x. This is not as easy as it might usually be, however. Mike Gunderloy gives some tips in case you get stuck.
Rails Security Guide - Steer clear of security issues in your Rails 2.2 applications by reading the Ruby on Rails Security Guide. Who said Rails has poor documentation? This is incredible!
Rails 2.2 Screencast - Gregg Pollack and Jason Seifer of Rails Envy put together a very solid Rails 2.2 screencast. It costs $9, but it covers a lot of ground over 44 minutes - learn about etags, connection pooling, new enumerable methods, new test helpers, and more.
Rails 2.2 - What's New - In association with EnvyCasts, Carlos Brando and Carl Youngblood present Rails 2.2 - What's New, a 118 page PDF covering all of the changes and additions to Rails 2.2. It's available in a package deal with the screencast (above) too.
InfoQ's Glance - InfoQ's Mirko Stocker takes a quick glance at some of Rails 2.2's new features.
New Features
Thread Safety - Rails 2.2 is now "thread safe." In October, Pratik Naik wrote a summary of why this is a big deal as well as some gotchas (basically, don't use class variables, use mutexes, etc.) Charles Nutter has also written What Thread-safe Rails Means which answers several pertinent questions.
Internationalization - The Rails Internationalization effort has its own homepage at http://rails-i18n.org/ which features lots of links to how-tos, tips, documentation, and demos. They also have a Google group / mailing list where you can get help, make suggestions, etc.
Basic Language Internationalization - It's a little old, but Simple Localization in Rails 2.2 gives a very quick, code-driven example of how basic internationalization works in Rails 2.2 (some of the set up is easier now, but it mostly applies).
Localization / Internationalization Demo App - Clemens Kofler has put together a demo app that shows off some of Rails 2.2's internationalization and localization features. If being knee deep in code is the best way for you to learn, jump in!
Layouts for ActionMailer - As of Rails 2.2, you can now use layouts in your ActionMailer views.
Connection Pooling - The connection pooling in Rails 2.2 allows Rails to distribute database requests across a pool of database connections. This can cause less lockups. In collaboration with a non-blocking MySQL driver, serious performance increases could result in certain situations.
Specify Join Table Conditions with Hashes - Do you need to run a find (or similar) query across a join? Now you can just specify the conditions for the joined tables in a hash, much like local tables conditions!
Limited Resource Routes - You can now limit map.resources to creating certain methods. For example, you might not want destroy or index methods - you can now specify these with :only and :except.
Memoization - Stop rolling your own memoization in Rails apps. Clemens Kofler demonstrates Rails 2.2's newly rolled-in memoization features. It's just a single method! If you have a view that calls on a calculated attribute often, this will give you some serious performance gains.
Custom Length Tokenizer for Validations - You can now specify a tokenizer of your own construction for validates_length_of validations.
Array#second through Array#tenth - If you're a bad programmer, you can now demonstrate it to the world by using the new Array#second, Array#third, Array#fourth, and so forth, methods. I've put it in my calendar to look for open source Rails apps using Array#seventh in six months time and to call them out on Rails Inside ;-)
Note: This list only takes into account some of the new features in Rails 2.2. There are a lot more! Read the release notes and the Rails 2.2 - What's New PDF to get the full picture.
Miscellaneous
restful-authentication-i18n - Want an authentication plugin for Rails 2.2 that supports internationalization? Take a look at result-authentication-i18n!
Barebones Apps - Check out Rails Inside's 7 Barebones Rails Apps to Kick Start Your Development Process.
Deploying on JBoss - You can now easily deploy a Rails app to a JBoss server. With Rails 2.2's significantly improved JRuby support, this makes rolling out Rails apps in the enterprise a breeze!
Installing Rails on Ubuntu Hardy Heron - Simon St Laurent has put together two Rails useful installation videos. One for servers, and one for the desktop.
REST for Rails 2 - Are you still in Rails 1.x land or not using REST at all? Would you like to? Geoffrey Grosenbach has put together a screencast showing you how it should be done.
A Better Rails Logo - The Rails Logo (as used at the head of this post) was created by Kevin Milden and is distrubuted under the BY-ND Creative Commons Licence. i personally like the older one.
Common RJS mistake/error on Ruby on Rails
When you use some of your ajax helpers, such as link_to_remote, with the :update parameter in order to update a specific DOM element AND you specify page.replace_html or any other RJS syntax that handles other DOM ID, it will return the result expected, nothing will be updated on the :update pointer DOM ID.
Simply choose one, if you use :update, return an html or render a partial in your action instead of rendering RJS.
If you require multiple changes, use RJS and no :update parameter.
Ruby on Rails and Oracle on Mac Os Leopard
Overview
The nightmare is over.
Just until the latest Oracle libraries update (finally released a X86 library pack for mac) it was nesecerry to use 2 versions of ruby, a universal and a ppc version. Sadly, when running PPC, the benchmarking were terrible and it had some very annoying freezes and other stuff that would simple make you want to jump off the roof.
BUT! (:) ) times had changed, Oracle (as mentioned) released an X86 Intel compatible library pack for MacOs users and therefore ended my misery,
Woohoo! That makes the entire process of connection Ruby on Rails and oracle on Leopard about as 100 times less complicated than before, so I’ve posted it here to let everyone enjoy.
I assumes that you’re using Rails 2.0 or greater (Why not really?).
IMPORTART!!!
If you already connected Oracle and Ruby on Rails using the old way, please preform the "Cleanup" step first.
Oracle Libraries
The new Intel Mac versions are available from the Oracle downloads site. Install them in /Library/Oracle/.
You can do side-by-side installations in folders with whatever names you want, since apps find them by using the $ORACLE_HOME environment variable (and it’s friends). I’ve got mine in /Library/Oracle/instantclient_10_2.
Also make sure that you’ve got the files required to run sqlplus and the sdk along with the basic. You can drop those in the same directory.
Symlink the libraries
In the directory where you’ve installed the instant client, run this:
ln -s libclntsh.dylib.10.1 libclntsh.dylib
Set the environment variables correctly
You’ll probably want to put these lines in your /etc/profile , but they also must be run from the command line to take effect (you can also "source /etc/profile"):
export ORACLE_HOME=/Library/Oracle/instantclient_10_2 <= Change to your library!
export TNS_ADMIN=$ORACLE_HOME
export LD_LIBRARY_PATH=$ORACLE_HOME
export DYLD_LIBRARY_PATH=$ORACLE_HOME
export PATH=$PATH:$ORACLE_HOME
Oracle! giddie up!
First you'll need to install the Active Record Oracle adapter,
sudo gem install activerecord-oracle-adapter --source http://gems.rubyonrails.org
which is how ActiveRecord deals with Oracle.
It doesn’t, however, install the Ruby oci8 driver, which is how Ruby talks to Oracle (yeah, annoying).
Important!!!
Have you installed the Oracle Instant Client SDK ?
good.
Get the lastest the oci8 library. Download it and unpack the file in the finder: it should unzip into ~/Downloads/ruby-oci8-x.x.x.
Now we can finish configuring the environment before we compile the library.
cd ~/Downloads/ruby-oci8-x.x.x
export SQLPATH=$ORACLE_HOME
export RC_ARCHS=i386
ruby setup.rb config
make
sudo make install
oh joy, scrolling lines of doom will pass in front of you and hopefully you'll see no errors and burst into tears.
Show me!
At this point, we’re almost done. Let's see it working.
irb
require 'oci8'
==> true
or
irb
require 'rubygems'
==> []
require 'oci8'
==> true
Configure your database.yml
In your database.yml, use the following to make it work:
development:
adapter: oracle
database: your_instance_name
username: your_user_name
password: your_password
The database name = > comes straight out of your tnsnames.ora file. You don’t need to specify any other connection information in database.yml, since the tnsnames.ora file has everything you need.
if you are using the oracle Express edition, it should look something like that.
development:
adapter: oci
host: //db_hostname:db_port/xe <== Oracle port is usually 1521
username: username
password: password
cursor_sharing: similar
prefetch_rows: 100
note: last 2 lines are some tweaking for a better Oracle performance.
Cleanup: Fix the ruby_fat and ruby_ppc setup
if you have installed Oracle libraries using the old way, you'll be happy to remove the mess it made out of your ruby installation and happily, it’s quite simple.
Just remove the ruby_ppc files, and the symlinks to them (called ruby, and rename ruby_fat as ruby:
cd /usr/bin <== or wherever you put them.
sudo rm ruby
sudo rm ruby_ppc
sudo mv ruby_fat ruby
and
cd /System/Library/Frameworks/Ruby.framework/Versions/1.8/usr/bin
sudo rm ruby
sudo rm ruby_ppc
sudo mv ruby_fat ruby
Then, you should also remove the 2 management scripts:
sudo rm /usr/bin/ppc_ruby.sh
sudo rm /usr/bin/fat_ruby.sh
And that’s enough for the cleanup.
TextMate Bundle of Bundles: getBundle
From Macroomates
There are 124 bundles for TextMate where only 36 are included by default. Until recently, the way to get more bundles has been to install subversion and then do a checkout of the bundles needed (from the shell.) or download a .tmbundle file.
Now there is a much easier way: the GetBundle bundle by Sebastian Gräßl. All you need to do is download and double click it to get an “Install Bundle” command which you can invoke from inside TextMate (hint: use ⌃⌘T and enter “install”.)
There is also an “Update all Bundles” command which you can use to update your custom installed bundles to the latest version. A future version of the bundle is likely to offer a launchd Daemon that you can install to have updating taken care of automatically.
All bundles installed via the GetBundle end up in ~/Library/Application Support/TextMate/Pristine Copy/Bundles.
Get it here, since the original repo isn't working.
Precentage proxy for Ruby's Numeric class
Just a little something i did, cause it usually takes me about 15 minutes to calculate a percentage conversion.
is anyone else checking/calculating dates in irb/console?
"Elad, in 2 weeks you have a dentist appointment!"
"mmm.... "
script/runner 'puts 2.weeks.from_now'
hehe,
but the method today's post is about, is the percent_from i did, so here it is.
class Numeric
def precent_from(num = 100)
self.to_f * (num.to_f / 100.0)
end
end
which comes out as:
>> 40.precent_from(1000)
=> 400.0
>> 6.precent_from(1000)
=> 60.0
Ruby on Rails 2.2 Release Notes
Rails 2.2 delivers a number of new and improved features. This list
covers the major upgrades, but doesn't include every little bug fix
and change. If you want to see everything, check out the list of
commits in the main Rails repository on GitHub.
Along with Rails, 2.2 marks the launch of the Ruby on Rails Guides,
the first results of the ongoing Rails Guides hackfest. This site will
deliver high-quality documentation of the major features of Rails.
Lighting Fast Ruby On Rails security checklist
Ruby on Rails Security checklist for models:
- Use attr_accessible (or attr_protected if you must) to explicitly identify attributes that are accessible by .create and .update_attributes. Just because you don't expose an attribute on an edit form doesn't mean that someone won't try to post a value to it. I prefer attr_accessible over attr_protected as it fails on the side of safety when new fields are added to a model - you have to explicitly expose new fields.
- Make sure queries are using the Rails bind variable facility for parameters, not string concatenation or the handy Ruby's #{...} syntax.
- Use validations to prevent bad input.
- Make non-action controller methods private (if possible).
- If non-action controller methods must be public, identify them with hide_action to prevent unwanted execution.
- Make sure before_filters are in place if necessary for your authorization infrastructure.
- Move queries from your controller to your model, and see the model checklist above.
- Check for params[:id] usage - are you sure you can trust it? Check for proper ownership of the record.
- Check for usage of hidden fields - a user can send anything to you through them, so treat them with suspicious just as params[:id] should be suspect.
- Use filter_parameter_logging to prevent entry of sensitive unencrypted data (passwords, SSN's, credit card numbers, etc.) in your server logs.
- Forget about your view code for a minute, and think about how to protect your controller from posts a malicious user could make to any of your exposed methods. All parameters (whether or not exposed on a form, and whether or not invisible) are suspect to length overruns, bypassing of any browser based validation, attacks with malformed data, etc.
- Make sure all data displayed is escaped with the helper method h(string).
- Eliminate comments in your views that you don't wish the entire world to see.
Buzz: rubyonrails.org vs. walla.co.il
Walla.co.il is one of the most visited web sites in Israel, it is some sort of an all-purpose-portal and holds the homepage spot for many people.
I wanted to check how many people are visiting in rubyonrails.org to walla.co.il at compete.com, this is what i got back.
Stand Alone ruby on rails application
Ok,
I Don't really know if anyone has ever done this before, but after viewing some unrelated posts regrading ruby and rails, i succeed in creating a stand alone ruby on rails application.
By saying stand alone, i mean you can copy your application to a usb driver, and start your mongrels anywhere you want!.
i wrote some scripts to setup what the application needs prior to loading, and got it running in no time.
i succeeded doing this with rails 1.2.6 so far, but i don't see any reason why i should not do it with 2.0/1.
now my offer goes a little of something like this, if you will come up with an idea how to make me (and you :) ) some money with this new trick, i'll give up 45%.
start thinking :)
Ruby on Rails Screen Casts (rated R :) )
I came across during my never-ending quest for Rails and Ruby related websites, in this cute site (re-design, fast) at Ruby Plus.
They composed over 90 (to this day) screen casts about ruby and rails, including some Rails 2.0 roundups, Ruby metaprogramming and so on.
use it, bookmark it, watch it. (and get the feed too :) ).
Ruby On Rails Developer Salary in the US
the graph is right here
Data Provided by of simplyhired.com, a search engine for jobs.
As i can see, $86,000 per year, that makes it about $7,100 USD a month, which is just a little more than what a super-rails ninja can get here in Israel, probably more for a short period projects.
how come?
Dump a Model table to fixtures
def self.to_fixture
write_file(File.expand_path("test/fixtures/#{table_name}.yml", RAILS_ROOT),
self.find(:all).inject("---\n") { |s, record|
self.columns.inject(s+"#{record.id}:\n") { |s, c|
s+" #{{c.name => record.attributes[c.name]}.to_yaml[5..-1]}\n" }
})
end
Twiters Around
About Me
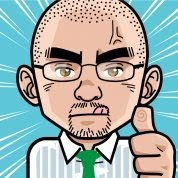
- Elad Meidar
- I am a web developer for more than 9 years, managed, cried, coded, designed and made money in this industry. now trying to do it again.
Blog Archive
-
▼
2009 (67)
-
▼
March (9)
- Moved to Mephisto
- Rails Nested Resources Tutorial
- Raphael: Amazing vector graphics Javascript Libraty
- Storing Files in Ruby on Rails
- Live Free: Open Source Software indexes
- Debugging Javascript in Web Applications: Major br...
- 140 Characters Web applications
- Rails and Facebook: Why (still) iFrame is better t...
- Rails Facebook App Enlightments: HTTP timeouts
-
▼
March (9)
Labels
- 1.9 (1)
- 2.0 (1)
- 2.2 (1)
- 2.3 (1)
- 2.x (1)
- accessibility (2)
- account (1)
- actionmailer (1)
- activerecord (3)
- adsense (1)
- affiliate (1)
- ajax (3)
- amazon (4)
- analytics (1)
- api (3)
- application (1)
- array (1)
- associations (1)
- attachment_fu (1)
- autocomplete (1)
- aws (2)
- blog (4)
- books (4)
- boolean (1)
- browser (8)
- browsers (3)
- bugs (4)
- buzz (1)
- callback (1)
- callbacks (1)
- caller (1)
- capistrano (1)
- chronic (1)
- class (2)
- classes (1)
- classifieds (1)
- client (1)
- coding (2)
- collboration (1)
- console (2)
- convert (1)
- core (2)
- core. object (1)
- cost (1)
- css (7)
- database (10)
- date (3)
- dating (1)
- db (2)
- debug (2)
- deploy (5)
- deployment (3)
- design (11)
- development (5)
- dojo (1)
- eager (1)
- ec2 (2)
- effects (1)
- elad (2)
- email (3)
- engine (1)
- engineyard (1)
- english (1)
- entrepreneur (1)
- environment (2)
- erd (1)
- error (1)
- example (1)
- expire (1)
- explorer (1)
- extention (1)
- extra (2)
- facebook (2)
- fast (1)
- file (1)
- files (1)
- firefox (4)
- fixtures (1)
- font (1)
- form (1)
- framework (16)
- free (6)
- funny (2)
- gem (7)
- gems (2)
- git (5)
- globalize (2)
- gmail (3)
- google (8)
- graphes (2)
- graphics (1)
- guides (4)
- hacks (1)
- hosting (1)
- html (1)
- http (1)
- ie (5)
- ie8 (1)
- image (2)
- indomite (1)
- install (1)
- iphone (1)
- israel (2)
- javascript (19)
- JQuery (10)
- js (3)
- legacy (1)
- leopard (1)
- lib (1)
- library (3)
- links (2)
- list (1)
- loading (1)
- log (1)
- logger (2)
- logging (1)
- love (1)
- mac (4)
- mail (2)
- manuals (1)
- mapping (1)
- mass (1)
- mephisto (3)
- mercurial (1)
- meta (1)
- metaprogramming (3)
- microsoft (1)
- migrations (2)
- minimagick (1)
- mistake (1)
- mistakes (1)
- model (1)
- money (4)
- mootools (4)
- move (1)
- movies (2)
- music (1)
- mvc (2)
- mysql (2)
- native (1)
- nested (1)
- network (1)
- new (1)
- newsletter (1)
- num_to_english (1)
- numbers (2)
- object (1)
- observer (1)
- ohad (1)
- open (1)
- operators (1)
- optimization (4)
- oracle (4)
- overload (1)
- parse (1)
- pdf (4)
- php (3)
- plugin (11)
- plugins (7)
- programming (4)
- programs (1)
- projects (5)
- prototype (1)
- queries (1)
- rails (50)
- rails 2.0 (10)
- rails 2.1 (6)
- rake (2)
- rants (1)
- record (1)
- reflections (1)
- release (3)
- rendering (1)
- reset (1)
- resize (1)
- rest (1)
- rjs (1)
- ror (1)
- routes (3)
- routes.rb (2)
- ruby (49)
- ruby on bells (2)
- ruby on rails (28)
- ruby-debug (1)
- s3 (2)
- salary (1)
- scaling (2)
- schema (2)
- screencasts (1)
- script (4)
- scripts (1)
- search engine optimization (1)
- security (3)
- seeking alpha (1)
- select (1)
- sendmail (1)
- seo (2)
- sequencer (1)
- sessions (1)
- share (2)
- shell (1)
- site (3)
- site map (1)
- sites (1)
- skype (1)
- social (2)
- source (1)
- source control (4)
- sprites (1)
- sql (1)
- stand alone (1)
- standards (1)
- startup (6)
- storage (2)
- subversion (4)
- svn (1)
- team (1)
- technology (1)
- testing (1)
- textmate (1)
- thread (1)
- time (1)
- tips (45)
- tools (5)
- tracking (1)
- tricks (36)
- tutorials (5)
- ui (4)
- uml (1)
- update (1)
- upgrading (2)
- url (1)
- usability (2)
- user interface (7)
- validations (1)
- vector (1)
- vote (1)
- web (9)
- web services (2)
- web2.0 (4)
- webistrano (1)
- wire (1)
- wwr (1)
- xhtml (1)
- yahoo (1)
- yui (1)
- רובי (8)
- רובי און ריילס (7)